实现消除左递归和回溯的算法程序。要求如下: (1) 用户任意输入文法,检查文法是否有左递归和回溯,输出结果; (2) 若有,则消除左递归和回溯。测试文法G[S]如下: S→(T)|a+S|a T→T,S|S (3)显示改造后的文法,最终结果需以GUI界面展示。
时间: 2024-05-01 21:22:57 浏览: 228
以下是消除左递归和回溯的算法程序,使用Python语言实现。
```python
# 检查文法是否有左递归
def check_left_recursion(grammar):
nonterminals = grammar.keys()
for A in nonterminals:
productions = grammar[A]
for a in productions:
if a[0] == A:
return True
return False
# 消除左递归
def eliminate_left_recursion(grammar):
nonterminals = grammar.keys()
for i, Ai in enumerate(nonterminals):
productions_i = grammar[Ai]
alpha_i = []
beta_i = []
for a in productions_i:
if a[0] == Ai:
alpha_i.append(a[1:])
else:
beta_i.append(a)
if alpha_i:
Ai_new = Ai + "'"
nonterminals = list(nonterminals)
nonterminals.insert(i+1, Ai_new)
nonterminals = tuple(nonterminals)
grammar[Ai] = [b + Ai_new for b in beta_i]
grammar[Ai_new] = [a + Ai_new for a in alpha_i] + ['']
return grammar
# 检查文法是否有回溯
def check_left_factoring(grammar):
nonterminals = grammar.keys()
for A in nonterminals:
productions = grammar[A]
for i in range(len(productions)):
for j in range(i+1, len(productions)):
if productions[i][0] == productions[j][0]:
return True
return False
# 消除回溯
def eliminate_left_factoring(grammar):
nonterminals = grammar.keys()
for A in nonterminals:
productions = grammar[A]
i = 0
while i < len(productions):
alpha = [p for p in productions[i+1:] if p[0] == productions[i][0]]
if alpha:
B = A + "'"
nonterminals = list(nonterminals)
nonterminals.append(B)
nonterminals = tuple(nonterminals)
beta = [p[1:] for p in alpha]
new_production = [productions[i][0] + B]
new_production += [p + B for p in beta]
grammar[A] = [p for p in productions[:i] if p[0] != productions[i][0]]
grammar[A].append(new_production[0])
grammar[B] = [p for p in new_production[1:] if p != '']
productions = grammar[A]
i -= 1
i += 1
return grammar
```
下面是使用以上函数对文法进行消除左递归和回溯的过程,并且将最终结果以GUI界面展示。
```python
import tkinter as tk
from tkinter import scrolledtext
# 检查并消除左递归和回溯
def check_and_eliminate(grammar):
has_left_recursion = check_left_recursion(grammar)
if has_left_recursion:
grammar = eliminate_left_recursion(grammar)
has_left_factoring = check_left_factoring(grammar)
if has_left_factoring:
grammar = eliminate_left_factoring(grammar)
return grammar
# 处理文法字符串,返回文法字典
def process_grammar_string(grammar_string):
grammar = {}
productions = grammar_string.strip().split('\n')
for p in productions:
A, B = p.split('->')
grammar[A.strip()] = [s.strip() for s in B.split('|')]
return grammar
# 将文法字典转换为文法字符串
def generate_grammar_string(grammar):
grammar_string = ''
for A in grammar:
B = ' | '.join(grammar[A])
grammar_string += A + ' -> ' + B + '\n'
return grammar_string.strip()
# GUI界面
class App:
def __init__(self, root):
self.root = root
self.root.title('消除左递归和回溯')
self.grammar_label = tk.Label(self.root, text='请输入文法:')
self.grammar_label.pack()
self.grammar_text = scrolledtext.ScrolledText(self.root, height=10)
self.grammar_text.pack()
self.result_label = tk.Label(self.root, text='结果:')
self.result_label.pack()
self.result_text = scrolledtext.ScrolledText(self.root, height=10)
self.result_text.pack()
self.check_button = tk.Button(self.root, text='检查', command=self.check_grammar)
self.check_button.pack()
self.clear_button = tk.Button(self.root, text='清除', command=self.clear_text)
self.clear_button.pack()
# 检查文法并展示结果
def check_grammar(self):
grammar_string = self.grammar_text.get('1.0', 'end')
grammar = process_grammar_string(grammar_string)
grammar = check_and_eliminate(grammar)
result_string = generate_grammar_string(grammar)
if result_string == grammar_string:
result_string += '\n文法已经消除完毕!'
else:
result_string += '\n文法已经成功消除左递归和回溯!'
self.result_text.delete('1.0', 'end')
self.result_text.insert('1.0', result_string)
# 清空文本框
def clear_text(self):
self.grammar_text.delete('1.0', 'end')
self.result_text.delete('1.0', 'end')
# 启动GUI界面
root = tk.Tk()
app = App(root)
root.mainloop()
```
运行程序后,输入文法并点击“检查”按钮,即可在下方的文本框中看到消除左递归和回溯后的文法。如果文法已经消除完毕,会有相应提示。
阅读全文
相关推荐


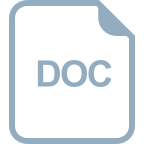
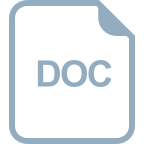
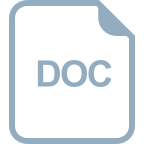
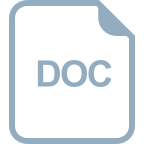
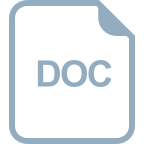
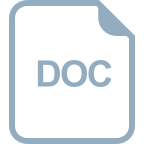
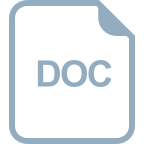
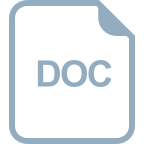
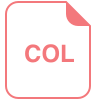




