Java从数组中删除重复项
时间: 2023-09-22 07:06:44 浏览: 70
### 回答1:
在 Java 中,可以使用以下步骤来从数组中删除重复项:
1. 定义一个 HashSet 对象,该对象不允许重复元素。
2. 遍历数组中的每个元素,并将其添加到 HashSet 中。如果元素已存在于 HashSet 中,则该元素是重复的,可以被忽略。
3. 使用 HashSet 的 toArray() 方法将其转换回数组。这将创建一个新的数组,其中不包含重复的元素。
下面是一个使用上述步骤的示例代码:
```
int[] array = {1, 2, 3, 4, 2, 3, 4, 5};
Set<Integer> set = new HashSet<>();
for (int i : array) {
set.add(i);
}
int[] result = new int[set.size()];
int i = 0;
for (int n : set) {
result[i++] = n;
}
```
在上面的代码中,result 数组将是一个不包含重复元素的新数组。
另外,也可以使用 Java 8 中的 Stream API 和 distinct() 方法来实现这个功能。例如:
```
int[] array = {1, 2, 3, 4, 2, 3, 4, 5};
int[] result = Arrays.stream(array).distinct().toArray();
```
这将返回一个新的 int 数组,其中包含原数组中的所有不重复元素。
希望这对你有帮助!
### 回答2:
在Java中删除一个数组中的重复项可以通过以下步骤实现:
1. 首先,创建一个新的空数组,用于存储没有重复项的元素。
2. 遍历原始数组中的每个元素。
3. 在遍历的过程中,使用一个布尔变量来判断当前元素是否已经在新数组中出现过。
4. 如果当前元素没有在新数组中出现过,则将其添加到新数组中。
5. 最后,返回新数组作为结果。
以下是一个示例代码:
```java
public static int[] removeDuplicates(int[] array) {
int[] newArray = new int[array.length];
int index = 0;
for (int i = 0; i < array.length; i++) {
boolean isDuplicate = false;
for (int j = 0; j < index; j++) {
if (array[i] == newArray[j]) {
isDuplicate = true;
break;
}
}
if (!isDuplicate) {
newArray[index] = array[i];
index++;
}
}
int[] result = new int[index];
System.arraycopy(newArray, 0, result, 0, index);
return result;
}
```
这个方法的时间复杂度为O(n^2),可以通过遍历原始数组中的每个元素,并在新数组中进行查找来确定是否为重复项。值得注意的是,这个方法会保留数组中首次出现的重复项,而将其他重复项删除。如果想要保留最后出现的重复项,可以逆序遍历原始数组,并逆序添加到新数组中。
### 回答3:
Java从数组中删除重复项的方法有很多种,以下是一种较为简单的实现方法:
1. 创建一个新的空数组,用于存储不重复的元素。
2. 使用for循环遍历原数组中的每个元素。
3. 在每次循环中,判断当前元素是否已经在新数组中存在。可以通过使用嵌套的for循环来遍历新数组,判断新数组中是否存在相同的元素。
4. 如果当前元素在新数组中不存在,则将其添加到新数组中。
5. 循环结束后,新数组中就存储了原数组中的不重复元素。
下面是一个示例代码:
```
public static int[] removeDuplicates(int[] arr) {
int[] newArr = new int[arr.length];
int index = 0; // 新数组的索引
for (int i = 0; i < arr.length; i++) {
boolean isDuplicate = false;
for (int j = 0; j < index; j++) {
if (arr[i] == newArr[j]) {
isDuplicate = true;
break;
}
}
if (!isDuplicate) {
newArr[index++] = arr[i];
}
}
int[] result = new int[index];
for (int i = 0; i < index; i++) {
result[i] = newArr[i];
}
return result;
}
```
使用该方法调用示例:
```
int[] arr = {1, 2, 3, 1, 2, 4, 5, 3};
int[] newArr = removeDuplicates(arr);
System.out.println(Arrays.toString(newArr)); // [1, 2, 3, 4, 5]
```
这样就能够从数组中删除重复项并返回一个不含重复元素的新数组。
相关推荐
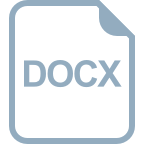














