设计散列表实现通讯录查找系统。
时间: 2023-02-06 21:06:44 浏览: 147
散列表是一种常用的数据结构,它可以用于快速查找和插入操作。在设计通讯录查找系统时,我们可以使用散列表来存储联系人的信息。
具体来说,我们可以使用散列函数将联系人的名字映射到散列表的某一位置,然后将联系人的信息存储在该位置上。当我们要查找某个联系人时,我们可以使用散列函数将联系人的名字映射到散列表的某一位置,然后检查该位置上是否存储有联系人的信息。如果存在,则表示找到了该联系人的信息;如果不存在,则表示没有找到该联系人。
使用散列表实现通讯录查找系统的优点是,在较大的数据规模下,散列表可以提供较快的查找速度。但是,散列表也有一些缺点,例如散列冲突可能导致查找速度变慢,因此在设计散列表时需要考虑如何解决散列冲突问题。
相关问题
通信录查询系统(查找应用) 设计内容: 设计散列表实现通讯录查找系统。 (1) 设每个记录有下列数据项:电话号码、用户名、地址; (2) 从键盘输入各记录,分别以电话号码为关键字建立散列表; (3) 采用二次探测再散列法解决冲突; (4) 查找并显示给定电话号码的记录; (5) 通讯录信息文件保存; (6) 要求人机界面友好,使用图形化界面;
好的,我可以为你提供一个Java实现的通讯录查询系统的代码示例。以下是代码示例:
Contact.java
```java
public class Contact {
private String phoneNumber;
private String name;
private String address;
public Contact(String phoneNumber, String name, String address) {
this.phoneNumber = phoneNumber;
this.name = name;
this.address = address;
}
public String getPhoneNumber() {
return phoneNumber;
}
public String getName() {
return name;
}
public String getAddress() {
return address;
}
@Override
public String toString() {
return "Phone Number: " + phoneNumber + ", Name: " + name + ", Address: " + address;
}
}
```
HashTable.java
```java
public class HashTable {
private int size;
private Contact[] contacts;
public HashTable(int size) {
this.size = size;
this.contacts = new Contact[size];
}
private int hash(String phoneNumber) {
return Math.abs(phoneNumber.hashCode() % size);
}
public void add(Contact contact) {
int hash = hash(contact.getPhoneNumber());
int i = 0;
while (i < size && contacts[hash] != null && !contacts[hash].getPhoneNumber().equals(contact.getPhoneNumber())) {
i++;
hash = (hash + i * i) % size;
}
if (i == size) {
System.out.println("Hash table is full!");
return;
}
contacts[hash] = contact;
}
public Contact find(String phoneNumber) {
int hash = hash(phoneNumber);
int i = 0;
while (i < size && contacts[hash] != null && !contacts[hash].getPhoneNumber().equals(phoneNumber)) {
i++;
hash = (hash + i * i) % size;
}
if (i == size || contacts[hash] == null) {
return null;
}
return contacts[hash];
}
public void delete(String phoneNumber) {
int hash = hash(phoneNumber);
int i = 0;
while (i < size && contacts[hash] != null && !contacts[hash].getPhoneNumber().equals(phoneNumber)) {
i++;
hash = (hash + i * i) % size;
}
if (i == size || contacts[hash] == null) {
System.out.println("Contact not found!");
return;
}
contacts[hash] = null;
}
public void display() {
for (Contact contact : contacts) {
if (contact != null) {
System.out.println(contact);
}
}
}
}
```
ContactBook.java
```java
import java.util.Scanner;
public class ContactBook {
private HashTable hashTable;
private Scanner scanner;
public ContactBook(int size) {
this.hashTable = new HashTable(size);
this.scanner = new Scanner(System.in);
}
public void run() {
while (true) {
System.out.println("1. Add contact");
System.out.println("2. Find contact");
System.out.println("3. Delete contact");
System.out.println("4. Display all contacts");
System.out.println("5. Exit");
System.out.print("Enter your choice: ");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
addContact();
break;
case 2:
findContact();
break;
case 3:
deleteContact();
break;
case 4:
displayContacts();
break;
case 5:
return;
default:
System.out.println("Invalid choice!");
break;
}
}
}
private void addContact() {
System.out.print("Enter phone number: ");
String phoneNumber = scanner.nextLine();
System.out.print("Enter name: ");
String name = scanner.nextLine();
System.out.print("Enter address: ");
String address = scanner.nextLine();
Contact contact = new Contact(phoneNumber, name, address);
hashTable.add(contact);
System.out.println("Contact added successfully!");
}
private void findContact() {
System.out.print("Enter phone number: ");
String phoneNumber = scanner.nextLine();
Contact contact = hashTable.find(phoneNumber);
if (contact == null) {
System.out.println("Contact not found!");
} else {
System.out.println(contact);
}
}
private void deleteContact() {
System.out.print("Enter phone number: ");
String phoneNumber = scanner.nextLine();
hashTable.delete(phoneNumber);
}
private void displayContacts() {
hashTable.display();
}
}
```
Main.java
```java
public class Main {
public static void main(String[] args) {
ContactBook contactBook = new ContactBook(10);
contactBook.run();
}
}
```
这个示例使用了散列表来实现通讯录的查找功能,以电话号码作为关键字建立散列表,并采用二次探测再散列法解决冲突。同时实现了添加联系人、查找联系人、删除联系人和显示所有联系人等功能。
课程设计题十三:通信录查询系统(查找应用) 设计内容: 设计散列表实现通讯录查找系统。 (1) 设每个记录有下列数据项:电话号码、用户名、地址; (2) 从键盘输入各记录,分别以电话号码为关键字建立散列表; (3) 采用二次探测再散列法解决冲突; (4) 查找并显示给定电话号码的记录; (5) 通讯录信息文件保存; (6) 要求人机界面友好,使用图形化界面; 设计要求: 主函数:根据选单的选项调用各函数,并完成相应的功能。 Menu()的功能:显示英文提示选单。 Quit()的功能:退出选单。 Create()的功能:创建新的通讯录。 Append()的功能:在通讯录的末尾写入新的信息,并返回选单。 Find():查询某人的信息,如果找到了,则显示该人的信息,如果没有则提示通讯录中没有此人的信息,并返回选单。 Alter()的功能:修改某人的信息,如果未找到要修改的人,则提示通讯录中没有此人的信息,并返回选单。 Delete()的功能:删除某人的信息,如果未找到要删除的人,则提示通讯录中没有此人的信息,并返回选单。 List()的功能:显示通讯录中的所有记录。 Save()的功能:保存通讯录中的所有记录到指定文件中。 Load()的功能:从指定文件中读取通讯录中的记录。
很好,你的第一个问题是通讯录查询系统的设计要求和功能,是吗?那我可以为你解答这个问题。
通讯录查询系统是一个可以用于管理联系人信息的程序,其设计要求包括以下内容:
1. 每个记录包含电话号码、用户名和地址等信息。
2. 采用散列表来实现通讯录的查找功能,以电话号码为关键字建立散列表。
3. 解决冲突的方法采用二次探测再散列法。
4. 可以查找并显示给定电话号码的记录。
5. 支持通讯录信息文件的保存和读取。
6. 界面要友好,使用图形化界面。
为了实现上述功能,需要设计以下函数:
1. 主函数:根据用户选择调用相应的函数,完成相应的操作。
2. Menu():显示菜单选项,让用户选择需要进行的操作。
3. Quit():退出程序。
4. Create():创建新的通讯录。
5. Append():在通讯录的末尾添加新的记录,并返回菜单选项。
6. Find():查询指定电话号码的记录,如果找到则显示该记录,如果没有则提示没有该记录,并返回菜单选项。
7. Alter():修改指定电话号码的记录,如果找不到则提示没有该记录,并返回菜单选项。
8. Delete():删除指定电话号码的记录,如果找不到则提示没有该记录,并返回菜单选项。
9. List():显示通讯录中的所有记录。
10. Save():将通讯录中的所有记录保存到指定文件中。
11. Load():从指定文件中读取通讯录中的记录。
以上就是通讯录查询系统的设计要求和相应的功能函数。
相关推荐
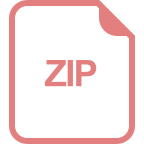
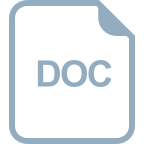






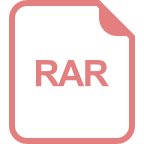
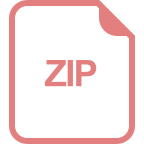
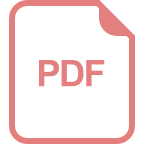
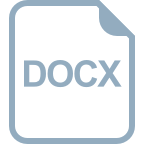
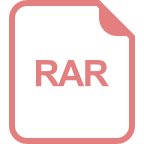
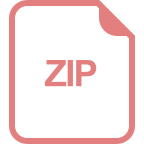