word2vec计算句子相似度的代码
时间: 2023-12-21 15:47:44 浏览: 26
以下是使用word2vec计算两个句子相似度的示例代码(使用Python和gensim库):
```python
from gensim.models import Word2Vec
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
# 加载预训练的word2vec模型
model = Word2Vec.load("path/to/word2vec/model")
# 定义函数计算两个句子的向量表示
def sentence_vector(sentence, model):
# 将句子分词并去掉停用词等无用词汇
words = [w for w in sentence.split() if w in model.wv.vocab]
# 如果句子中所有词汇都不在词向量模型中,则返回0向量
if len(words) == 0:
return np.zeros(300)
# 计算句子中所有词汇的词向量表示的平均值作为句子的向量表示
else:
return np.mean(model[words], axis=0)
# 计算两个句子的向量表示
sentence1 = "Hello world"
sentence2 = "Hi there"
vector1 = sentence_vector(sentence1, model)
vector2 = sentence_vector(sentence2, model)
# 计算两个句子的余弦相似度
similarity = cosine_similarity([vector1], [vector2])[0][0]
print("Similarity between '{}' and '{}': {}".format(sentence1, sentence2, similarity))
```
上述代码中,我们首先加载了预训练的word2vec模型。然后定义了一个函数`sentence_vector`,用于计算一个句子的向量表示。该函数首先将句子分词并去掉停用词等无用词汇,然后计算句子中所有词汇的词向量表示的平均值作为句子的向量表示。如果句子中所有词汇都不在词向量模型中,则返回0向量。接下来,我们使用`sentence_vector`函数计算了两个句子的向量表示,并使用`cosine_similarity`函数计算了它们的余弦相似度。最后,我们将计算结果打印出来。
需要注意的是,上述代码中的词向量维度为300,如果你的word2vec模型中的词向量维度不同,需要相应地修改代码。
相关推荐
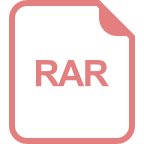
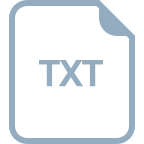
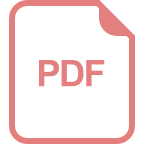












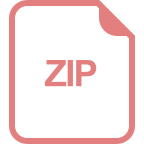
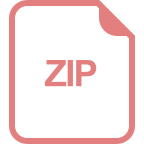