java编程实现投票程序,要求如下:定义一个Candidate类,类中有两个属性name和count程序读入candidate.txt中候选人名单票数排序后,屏幕降序显示投票结果。将投票结果保存到result
时间: 2024-12-06 10:27:14 浏览: 16
在Java编程中,你可以按照以下步骤创建一个简单的投票程序:
首先,定义一个`Candidate`类,用于表示候选人,包含姓名(name)和得票数(count):
```java
public class Candidate {
private String name;
private int count;
public Candidate(String name) {
this.name = name;
this.count = 0; // 初始化得票数为0
}
public String getName() {
return name;
}
public void incrementCount() {
count++;
}
@Override
public String toString() {
return name + " - " + count + " votes";
}
}
```
然后,读取`candidate.txt`文件,这个文件应该是一行一个候选人的名字格式,例如:
```
Alice
Bob
Charlie
David
...
```
你可以使用`BufferedReader`来逐行读取文件内容:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
List<Candidate> candidates = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader("candidate.txt"))) {
String line;
while ((line = br.readLine()) != null) {
candidates.add(new Candidate(line));
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
```
接着对候选人列表进行排序。这里我们按得票数降序排列:
```java
Collections.sort(candidates, Collections.reverseOrder(Comparator.comparing(Candidate::getCount)));
```
最后,遍历并打印投票结果,并将其保存到`result.txt`文件中:
```java
try (BufferedWriter bw = new BufferedWriter(new FileWriter("result.txt"))) {
for (Candidate candidate : candidates) {
bw.write(candidate.toString() + "\n");
}
bw.flush();
} catch (IOException e) {
System.err.println("Error writing to result file: " + e.getMessage());
}
System.out.println("Votes results:");
for (Candidate candidate : candidates) {
System.out.println(candidate);
}
```
完整的`Main`类可能会长一些,但核心就是以上部分。记得处理可能出现的异常,并在实际项目中添加适当的错误处理。
阅读全文
相关推荐
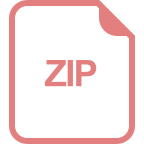
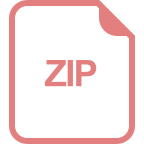
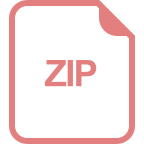















