mybatisplus中两表联查并且用第三个实体类存储查询结果
时间: 2023-11-25 08:44:45 浏览: 66
在 MyBatis Plus 中进行两表联查并使用第三个实体类存储查询结果,可以使用 MyBatis Plus 提供的 `@TableField` 注解来映射查询结果到实体类的字段。
假设有以下三个实体类:
```java
@TableName("notification")
public class Notification {
@TableId(value = "id", type = IdType.AUTO)
private Long id;
@TableField("title")
private String title;
// 其他字段...
// Getter 和 Setter 方法省略...
}
@TableName("student_notification")
public class StudentNotification {
@TableField("student_sno")
private String studentSno;
@TableField("is_show")
private Integer isShow;
// 其他字段...
// Getter 和 Setter 方法省略...
}
public class NotificationInfo {
private Notification notification;
private StudentNotification studentNotification;
// Getter 和 Setter 方法省略...
}
```
首先,创建一个新的实体类 `NotificationInfo`,其中包含了 `Notification` 和 `StudentNotification` 两个实体类作为字段,并使用 `@TableField` 注解进行映射。
然后,使用 MyBatis Plus 的查询构造器来进行联表查询,并将查询结果映射到 `NotificationInfo` 实体类。示例代码如下:
```java
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class NotificationService {
@Autowired
private NotificationMapper notificationMapper;
public List<NotificationInfo> getNotificationInfoList() {
QueryWrapper<NotificationInfo> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("student_notification.is_show", 0)
.eq("notification.id", new SqlSelect().column("notification_id").from("student_notification"))
.eq("notification.id", new SqlSelect().column("id").from("notification"));
return notificationMapper.getNotificationInfoList(queryWrapper);
}
}
```
上述代码中,我们使用 `QueryWrapper` 构建查询条件,并在 `NotificationMapper` 中定义了 `getNotificationInfoList()` 方法来执行联表查询。具体的实现可以参考以下代码:
```java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface NotificationMapper extends BaseMapper<Notification> {
List<NotificationInfo> getNotificationInfoList(@Param(Constants.WRAPPER) Wrapper<NotificationInfo> queryWrapper);
}
```
在 `NotificationMapper` 中,我们通过 `getNotificationInfoList()` 方法来执行联表查询,并将查询结果映射到 `NotificationInfo` 实体类。
需要注意的是,在 MyBatis Plus 中进行两表联查并使用第三个实体类存储查询结果时,需要保证实体类的字段与查询结果的字段一一对应,以便正确映射结果。
希望以上信息能对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
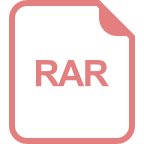
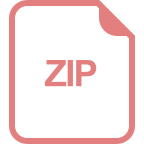
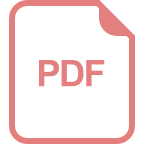














