使用C语言,完成下列题目。 设ha=(a1,a2,…,an)和 hb=(b1,b2,…,bm)是两个带头结点的循环单链表,ha和hb是尾指针,分别指向ha和hb两个循环单链表中的最后一个结点,设计一个算法将这两个表合并为带头结点的循环单链表hc,hc是尾指针
时间: 2024-11-12 13:46:09 浏览: 20
要在C语言中合并两个循环单链表,首先需要了解链表的基本结构和操作。假设链表节点有三个部分:数据域、下一个节点指针以及头节点指针(如果是单链表)。以下是合并两个循环链表的一个步骤描述:
1. 初始化新链表hc,其头节点设置为第一个列表ha的头节点。
2. 检查两个链表是否为空。如果其中一个为空,则将另一个链表直接作为结果返回。
3. 当ha和hb都非空时,遍历两个链表:
a. 比较当前节点的数据值,选择较小的那个放入新的链表hc。
b. 将较小的节点移到hc的头部,并更新hc的头指针。
c. 更新ha或hb的指针,使其指向当前节点的下一个节点。
d. 如果ha或hb已经到达它们各自的最后一个节点,那么将另一个链表的剩余部分添加到hc的尾部。
4. 合并完成后,将hc的尾指针指向ha或hb的原尾指针,因为它们原本就是循环链表的结尾。
下面是简化版的伪代码示例:
```c
struct ListNode {
int data;
struct ListNode* next;
struct ListNode* tail;
};
void mergeCircularLists(struct ListNode* ha, struct ListNode* hb, struct ListNode** hc) {
*hc = ha; // Set the head of new list to the head of first one
if (ha == NULL || hb == NULL) return;
while (true) {
if (ha->data <= hb->data) {
(*hc)->next = ha;
ha = ha->tail;
} else {
(*hc)->next = hb;
hb = hb->tail;
}
// If we reach the end of either list, append the other's remaining part
if (ha == ha->tail && hb != hb->tail) {
(*hc)->tail->next = hb;
*hc = hb->tail;
} else if (hb == hb->tail && ha != ha->tail) {
(*hc)->tail->next = ha;
*hc = ha->tail;
}
// If both pointers point to the same node, we have merged completely
if (ha == hb) break;
}
}
```
阅读全文
相关推荐
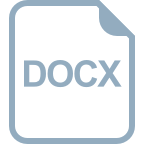
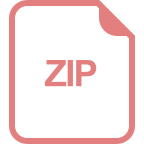
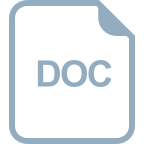
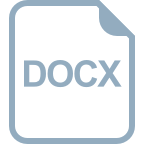
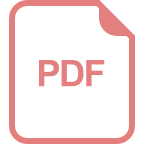
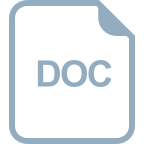
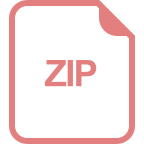
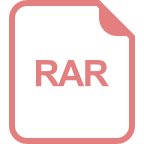
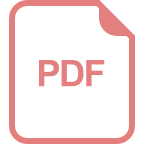
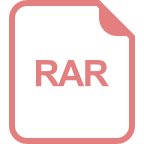
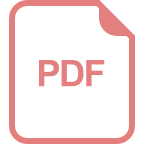
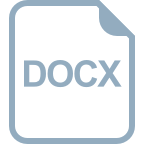
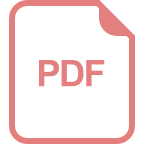
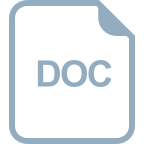