前后端项目分离,如何实现批量删除操作,vue文件中如何写前端,idea中mapper、biz、controller该如何写代码
时间: 2024-09-13 18:14:42 浏览: 67
在前后端分离的项目中实现批量删除操作,通常需要前端发送批量删除的请求给后端,后端接收到请求后执行删除逻辑,然后返回操作结果给前端。下面分别从前端Vue文件的写法和后端在IntelliJ IDEA中的代码编写进行说明。
### Vue文件中的前端实现
在Vue组件中,你可以使用axios或其他HTTP库来发送删除请求。这里以axios为例,假设后端提供了一个`/api/items/batchDelete`的接口用于处理批量删除,前端代码可能如下所示:
```vue
<template>
<div>
<!-- 这里可以是表格或其他列表展示,提供选择复选框 -->
<ul>
<li v-for="item in items" :key="item.id">
<input type="checkbox" v-model="selectedIds" :value="item.id" />
{{ item.name }}
</li>
</ul>
<button @click="handleBatchDelete">批量删除</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
selectedIds: [], // 存储选中的项的ID
items: [] // 初始数据列表
};
},
methods: {
async fetchItems() {
// 假设你有一个获取列表的函数
try {
const { data } = await axios.get('/api/items');
this.items = data;
} catch (error) {
console.error(error);
}
},
handleBatchDelete() {
if (this.selectedIds.length === 0) {
alert('请选择需要删除的项');
return;
}
axios.delete('/api/items/batchDelete', { data: this.selectedIds })
.then(response => {
// 处理响应结果
console.log(response.data);
// 可能需要根据后端返回的数据决定是否刷新列表等操作
this.fetchItems(); // 重新获取更新后的列表
})
.catch(error => {
console.error(error);
});
}
},
mounted() {
this.fetchItems(); // 组件加载时获取数据列表
}
};
</script>
```
### IntelliJ IDEA中后端代码编写
在IntelliJ IDEA中,你需要按照MVC模式编写对应的mapper、biz、controller代码。
#### Mapper层(MyBatis示例)
```java
public interface ItemMapper {
int deleteBatch(List<Long> ids);
}
```
对应的XML映射文件可能如下:
```xml
<mapper namespace="com.yourpackage.mapper.ItemMapper">
<delete id="deleteBatch">
DELETE FROM item WHERE id IN
<foreach item="id" collection="list" open="(" separator="," close=")">
#{id}
</foreach>
</delete>
</mapper>
```
#### Biz层
```java
public interface ItemService {
boolean batchDelete(List<Long> ids);
}
```
实现类:
```java
@Service
public class ItemServiceImpl implements ItemService {
@Autowired
private ItemMapper itemMapper;
@Override
public boolean batchDelete(List<Long> ids) {
int result = itemMapper.deleteBatch(ids);
return result > 0;
}
}
```
#### Controller层
```java
@RestController
@RequestMapping("/api/items")
public class ItemController {
@Autowired
private ItemService itemService;
@DeleteMapping("/batchDelete")
public ResponseEntity<?> batchDelete(@RequestBody List<Long> ids) {
boolean result = itemService.batchDelete(ids);
if (result) {
return ResponseEntity.ok().build();
} else {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
```
阅读全文
相关推荐
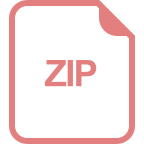
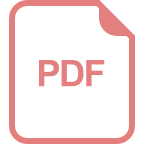
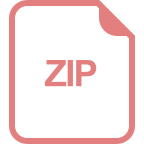










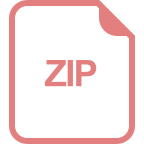
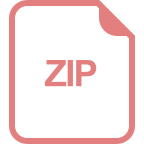
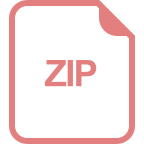
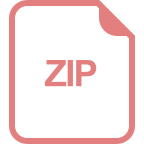
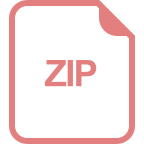