cpp中怎么记录数组中重复的个数
时间: 2024-12-14 14:16:14 浏览: 1
在C++中,记录数组中重复元素及其出现次数的一种常见方法是使用哈希表(unordered_map 或 unordered_set)。哈希表可以在常数时间内查找元素并更新计数。以下是使用`std::unordered_map`的一个示例:
```cpp
#include <iostream>
#include <unordered_map>
int countDuplicates(int arr[], int n) {
std::unordered_map<int, int> countMap;
for (int i = 0; i < n; ++i) {
// 如果元素已经在map中,则增加计数,否则添加新元素并设置计数为1
countMap[arr[i]] += (countMap.find(arr[i]) != countMap.end()) ? 1 : 0;
}
// 现在遍历map,输出重复元素及其个数
for (const auto &pair : countMap) {
if (pair.second > 1) {
std::cout << "元素 " << pair.first << " 重复次数: " << pair.second << "\n";
}
}
return 0;
}
int main() {
int arr[] = {1, 2, 3, 2, 4, 5, 6, 2};
int n = sizeof(arr) / sizeof(arr[0]);
countDuplicates(arr, n);
return 0;
}
```
在这个例子中,`countDuplicates`函数接收一个整数数组和它的长度,然后通过遍历数组并将每个元素的值作为键存储到哈希表中,值则是该元素出现的次数。最后,遍历哈希表并打印出重复的元素及其计数。
阅读全文
相关推荐
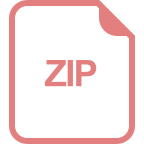
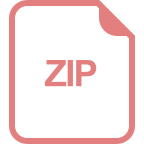
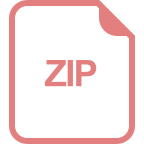














