模拟退火算法求线性规划的最大值怎么用代码实现
时间: 2024-09-07 22:03:03 浏览: 55
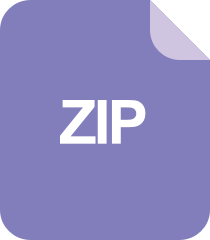
模拟退火算法matlab代码.zip
模拟退火算法是一种启发式搜索算法,用于解决优化问题。它是受物理中固体退火过程的启发,通过模拟物质加热后再缓慢冷却的过程,以此来找到系统的最低能量状态,即问题的最优解。在求解线性规划的最大值问题时,模拟退火可以用来寻找目标函数的最大值。
以下是使用模拟退火算法求解线性规划最大值问题的简化步骤:
1. 定义目标函数:在问题中找到需要最大化或最小化的函数,将其定义为模拟退火的目标函数。
2. 初始化参数:设定初始解、初始温度、冷却速率、终止温度等参数。
3. 随机扰动:从初始解出发,对当前解进行随机扰动,产生新的解。
4. 接受准则:根据目标函数的值和温度确定是否接受新解。在高温时,即使新解不是最优解,也有一定概率接受它,以避免陷入局部最优解。
5. 更新温度:根据冷却计划降低系统温度。
6. 终止条件:当温度低于预设的终止温度,或者达到一定的迭代次数时停止迭代。
7. 输出最优解:算法终止后,输出当前最优解。
以下是一个使用Python实现线性规划最大值问题模拟退火算法的代码框架:
```python
import numpy as np
# 定义目标函数
def objective_function(x):
# 这里以最大化线性函数 3x + 4y 为例
return 3*x + 4*y
# 生成随机解
def generate_random_solution():
# 这里需要根据实际问题来生成合法的解
return np.random.rand(2)
# 扰动解
def perturb_solution(solution):
# 扰动解并确保结果合法
solution[0] += np.random.randn() / 10
solution[1] += np.random.randn() / 10
return solution
# 更新温度
def update_temperature(temperature, cooling_rate):
return temperature * cooling_rate
# 判断新解是否更优
def accept_new_solution(old_value, new_value, temperature):
if new_value > old_value:
return True
else:
probability = np.exp((new_value - old_value) / temperature)
return np.random.rand() < probability
# 模拟退火算法主函数
def simulated_annealing():
current_solution = generate_random_solution()
current_value = objective_function(current_solution)
temperature = 1.0
cooling_rate = 0.995
while temperature > 1e-3:
new_solution = perturb_solution(current_solution)
new_value = objective_function(new_solution)
if accept_new_solution(current_value, new_value, temperature):
current_solution = new_solution
current_value = new_value
temperature = update_temperature(temperature, cooling_rate)
return current_solution, current_value
# 执行模拟退火算法
best_solution, best_value = simulated_annealing()
print(f"最优解:{best_solution}, 最优值:{best_value}")
```
请注意,这个代码仅提供一个基本框架,具体实现时需要根据线性规划的具体形式和约束条件进行相应的调整。在实际应用中,可能需要对解的生成、扰动、接受准则等环节进行更精细的控制。
阅读全文
相关推荐
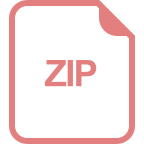
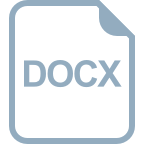
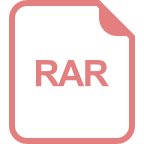
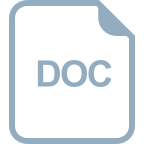
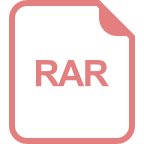
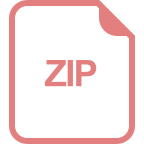
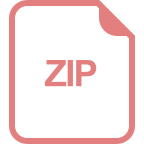
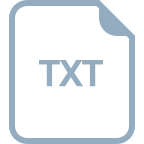
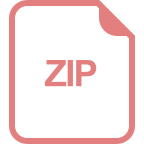
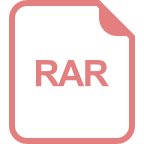
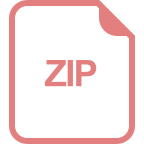
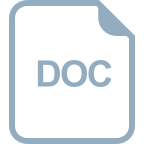
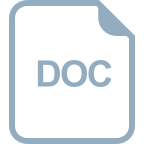
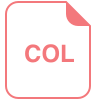
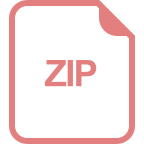