编写一个人事管理系统代码,运用链表,实现员工信息录入,修改且有输入文件和输出文件
时间: 2024-09-12 07:13:04 浏览: 46
创建一个人事管理系统,我们可以使用C++中的结构体来表示员工信息,链表(如单链表或双向链表)来存储这些信息,同时利用文件I/O来实现数据的持久化。这里是一个简单的示例,演示如何使用单链表和文本文件进行操作:
首先,定义一个Employee结构体,包含姓名、工号和职位等字段:
```cpp
struct Employee {
string name;
int id;
string position;
struct Node *next; // 链表节点,指向下一个员工
};
```
然后,定义链表操作相关的函数:
1. 插入新员工到链表末尾:
```cpp
void insert(Employee *&head, const Employee &newEmp) {
if (head == nullptr) {
head = new Employee{newEmp.name, newEmp.id, newEmp.position, nullptr};
} else {
Employee *current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Employee{newEmp.name, newEmp.id, newEmp.position, nullptr};
}
}
```
2. 修改员工信息:
```cpp
void update(Employee **node, const Employee &newEmp) {
if (*node != nullptr && (*node)->id == newEmp.id) {
(*node)->name = newEmp.name;
(*node)->position = newEmp.position;
}
}
```
3. 读取并显示链表中的所有员工:
```cpp
void displayList(const Employee *head) {
if (head == nullptr) {
cout << "No employees in the list.\n";
} else {
while (head != nullptr) {
cout << "ID: " << head->id << ", Name: " << head->name << ", Position: " << head->position << endl;
head = head->next;
}
}
}
```
接下来,处理文件操作:
1. 从文件读取员工信息并插入链表:
```cpp
void loadFromFile(Employee *&head, const string &filename) {
ifstream file(filename);
if (file.is_open()) {
string line;
while (getline(file, line)) {
stringstream ss(line);
Employee newEmp;
getline(ss, newEmp.name, ',');
ss >> newEmp.id;
getline(ss, newEmp.position, '\n');
insert(head, newEmp);
}
file.close();
} else {
cerr << "Unable to open the file." << endl;
}
}
```
2. 将链表保存到文件:
```cpp
void saveToFile(const Employee *head, const string &filename) {
ofstream file(filename);
if (file.is_open()) {
for (const Employee *emp = head; emp != nullptr; emp = emp->next) {
file << emp->name << "," << emp->id << "," << emp->position << endl;
}
file.close();
} else {
cerr << "Unable to open the file for writing." << endl;
}
}
```
现在你可以根据需求组合这些函数,例如在主程序中先加载数据,然后添加/修改员工,最后保存到文件。记得在每个操作后检查文件是否成功打开和关闭。
阅读全文
相关推荐
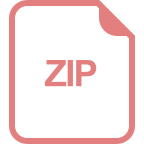
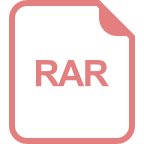
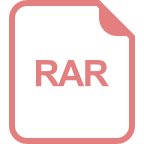



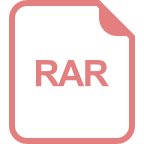
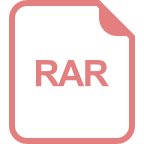
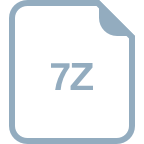
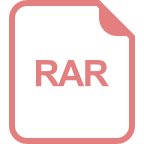
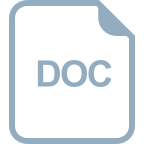
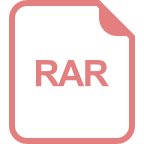
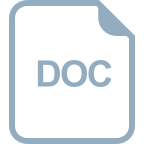
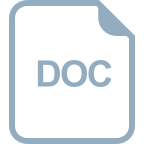
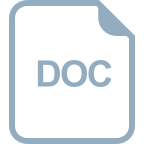
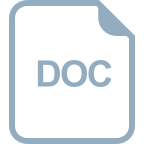
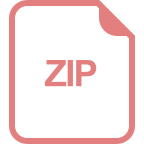
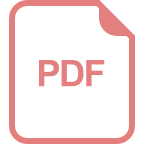