来一段手势python代码
时间: 2023-09-22 10:06:46 浏览: 101
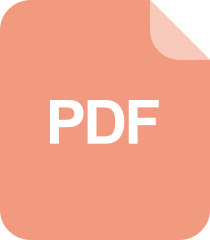
python实现手势识别的示例(入门)
### 回答1:
好的,这是一段用于识别手势的简单 Python 代码:
```
import cv2
import numpy as np
def detect_gesture(frame):
# 将图像转换为灰度图
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 设置阈值,将图像二值化
ret, thresh = cv2.threshold(gray, 150, 255, cv2.THRESH_BINARY)
# 检测图像中的轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 如果未检测到轮廓,则返回 None
if not contours:
return None
# 寻找最大轮廓
cnt = max(contours, key=cv2.contourArea)
# 计算轮廓的凸包
hull = cv2.convexHull(cnt)
# 计算凸包上的中心
moments = cv2.moments(cnt)
center = (int(moments['m10'] / moments['m00']), int(moments['m01'] / moments['m00']))
# 如果凸包上的中心位于图像中心,则返回 "open",否则返回 "closed"
if center[1] < thresh.shape[0] // 2:
return "open"
else:
return "closed"
```
这段代码使用 OpenCV 库识别图像中的手势。首先,图像被转换为灰度图,然后通过阈值将图像二值化。接下来,代码检测图像中的轮廓,并寻找其中面积最大的轮廓。最后,代码计算该轮廓的凸包并确定凸包上的中心,如果该中心位于图像中心以
### 回答2:
当然可以!以下是一个用手势控制的简单Python代码示例:
```python
import cv2
import mediapipe as mp
import math
mp_drawing = mp.solutions.drawing_utils
mp_hands = mp.solutions.hands
# 初始化视频捕获对象
cap = cv2.VideoCapture(0)
# 初始化手部检测模型
with mp_hands.Hands(min_detection_confidence=0.5, min_tracking_confidence=0.5) as hands:
while cap.isOpened():
# 读取视频帧
success, image = cap.read()
if not success:
print("无法读取视频帧")
break
# 将图像从BGR格式转换为RGB格式
image = cv2.cvtColor(cv2.flip(image, 1), cv2.COLOR_BGR2RGB)
# 设置图像输入
image.flags.writeable = False
results = hands.process(image)
# 绘制手部关键点及连接线
image.flags.writeable = True
image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR)
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
mp_drawing.draw_landmarks(image, hand_landmarks, mp_hands.HAND_CONNECTIONS)
# 获取手部关键点坐标
for land_id, lm in enumerate(hand_landmarks.landmark):
height, width, _ = image.shape
cx, cy = int(lm.x * width), int(lm.y * height)
# 根据手部关键点坐标进行手势判断
# 在这里编写你的手势判断代码
# 显示图像
cv2.imshow("Gesture Recognition", image)
# 按下'q'键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
需要注意的是,这只是一个示例代码,无法判断手势。在注释标注的位置,你可以根据手部关键点坐标进行自定义的手势判断,并实现相应的功能逻辑。
### 回答3:
当然,请看下面的代码:
```python
import cv2
import mediapipe as mp
# 使用mediapipe加载Hand模型
mp_drawing = mp.solutions.drawing_utils
mp_hands = mp.solutions.hands
# 从摄像头获取实时视频流
cap = cv2.VideoCapture(0)
with mp_hands.Hands(
max_num_hands=1,
min_detection_confidence=0.5,
min_tracking_confidence=0.5) as hands:
while cap.isOpened():
ret, frame = cap.read()
if not ret:
print("无法读取视频流.")
break
# 水平翻转图像,使镜像更符合人眼习惯
frame = cv2.flip(frame, 1)
# 将图像转换为RGB以供mediapipe使用
image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image.flags.writeable = False
# 处理图像,检测手部
results = hands.process(image)
# 渲染手部关键点和连接线
image.flags.writeable = True
image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR)
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
mp_drawing.draw_landmarks(
image, hand_landmarks, mp_hands.HAND_CONNECTIONS)
# 显示结果图像
cv2.imshow('Hand Gestures', image)
if cv2.waitKey(10) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这段代码使用`mediapipe`库中的`Hands`模型来检测摄像头实时视频中的手部,并将检测结果实时显示在窗口中。可以根据检测到的手部关键点来识别不同的手势,例如拇指向上、手掌打开等。
阅读全文
相关推荐
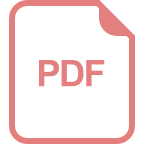
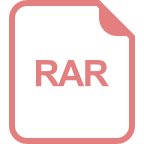






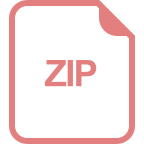
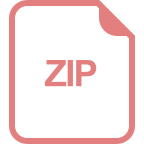
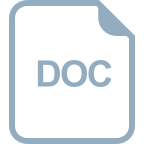






