请给我手势识别的具体python代码
时间: 2023-09-10 12:05:03 浏览: 64
### 回答1:
我可以提供一段简单的Python代码来展示手势识别的过程:import cv2# Load the cascade
hand_cascade = cv2.CascadeClassifier('hand_haar_cascade.xml')# Read the input image
img = cv2.imread('hand.jpg')# Convert into grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)# Detect hands in the image
hands = hand_cascade.detectMultiScale(gray, 1.1, 4)# Draw rectangle around the hands
for (x, y, w, h) in hands:
cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2)# Display the output
cv2.imshow('img', img)
cv2.waitKey()
### 回答2:
手势识别是一种通过计算机视觉技术来识别和理解人类手势的方法。下面是一个简单的基于Python与OpenCV库实现的手势识别代码示例:
首先,你需要安装OpenCV库,并导入所需的模块:
```python
import cv2
import numpy as np
import math
```
然后,你需要加载训练好的手势分类器文件(.xml格式):
```python
gesture_classifier = cv2.CascadeClassifier('gesture_classifier.xml')
```
接下来,你需要打开摄像头并读取视频流:
```python
capture = cv2.VideoCapture(0)
while True:
ret, frame = capture.read()
```
然后,将手势分类器应用于每一帧图像:
```python
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
gestures = gesture_classifier.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(100, 100))
```
在检测到手势后,你可以在图像上绘制矩形框标记手势位置:
```python
for (x, y, w, h) in gestures:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
```
为了实现更高级的手势识别,你可以检测手的轮廓并计算手指数量等手势特征:
```python
roi_gray = gray[y:y+h, x:x+w]
roi_color = frame[y:y+h, x:x+w]
blurred = cv2.GaussianBlur(roi_gray, (35, 35), 0)
_, threshold = cv2.threshold(blurred, 127, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
contours, _ = cv2.findContours(threshold, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
if len(contours) > 0:
contour = max(contours, key=lambda x: cv2.contourArea(x))
hull = cv2.convexHull(contour)
defects = cv2.convexityDefects(contour, hull)
finger_count = 0
for i in range(defects.shape[0]):
start, end, far, _ = defects[i, 0]
start_point = tuple(contour[start][0])
end_point = tuple(contour[end][0])
far_point = tuple(contour[far][0])
a = math.sqrt((end_point[0] - start_point[0])**2 + (end_point[1] - start_point[1])**2)
b = math.sqrt((far_point[0] - start_point[0])**2 + (far_point[1] - start_point[1])**2)
c = math.sqrt((end_point[0] - far_point[0])**2 + (end_point[1] - far_point[1])**2)
angle = math.acos((b**2 + c**2 - a**2) / (2*b*c)) * (180 / math.pi)
if angle <= 90:
finger_count += 1
cv2.putText(frame, f"Fingers: {finger_count}", (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
```
最后,你可以显示处理后的图像并等待用户退出:
```python
cv2.imshow("Gesture Recognition", frame)
if cv2.waitKey(1) == ord('q'):
break
```
完成所有代码后,你可以运行程序并在摄像头捕捉的图像上进行实时手势识别。
请注意,以上代码只是一个简单的手势识别示例,如果你想实现更复杂的手势识别,还需要进行更多的图像处理和算法优化。
相关推荐
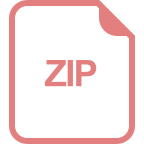
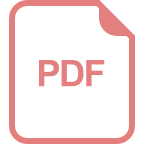














