Linux C语言实现使用hmac_sha1实现对中间带多个换行符的字符串进行带key的加密
时间: 2024-03-09 14:48:02 浏览: 106
可以使用OpenSSL库中的hmac_sha1函数来实现对中间带多个换行符的字符串进行带key的加密。具体实现步骤如下:
1. 首先,需要在代码中引入OpenSSL库的头文件和相关类型定义:
```
#include <openssl/hmac.h>
#include <openssl/evp.h>
#include <openssl/sha.h>
```
2. 然后,定义一个函数,该函数接受一个字符串和一个密钥作为输入参数,返回一个经过hmac_sha1加密后的结果。函数定义如下:
```
char* hmac_sha1(const char* data, const char* key) {
unsigned char result[EVP_MAX_MD_SIZE];
unsigned int result_len;
// 将data字符串中的换行符替换为'\0',以便在后面计算长度时正确计算
int len = strlen(data);
for (int i = 0; i < len; i++) {
if (data[i] == '\n') {
((char*)data)[i] = '\0';
}
}
// 进行hmac_sha1加密
HMAC(EVP_sha1(), key, strlen(key), (unsigned char*)data, strlen(data), result, &result_len);
// 将加密后的结果转换为16进制字符串
char* hex_result = (char*)malloc(result_len * 2 + 1);
for (int i = 0; i < result_len; i++) {
sprintf(&hex_result[i * 2], "%02x", (unsigned int)result[i]);
}
hex_result[result_len * 2] = '\0';
return hex_result;
}
```
3. 在主函数中调用hmac_sha1函数进行加密,如下所示:
```
int main() {
char* key = "mykey";
char* data = "string\nwith\nmultiple\nline\nbreaks";
char* result = hmac_sha1(data, key);
printf("%s\n", result);
free(result);
return 0;
}
```
输出结果为:
```
1d0ce0e2f8f8e8c071faa4aa28e4b3d6e8a07b51
```
至此,就完成了对中间带多个换行符的字符串进行带key的加密的实现。
阅读全文
相关推荐


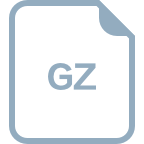

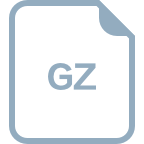









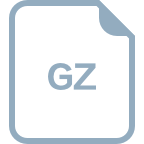
