写一个用TensorFlow 实现的transfomer,从定义超参数开始
时间: 2023-03-02 13:54:41 浏览: 58
在TensorFlow中实现Transformer模型,需要先定义一些超参数。超参数是指模型不能从数据中学习的参数,需要人为设定的参数。常见的Transformer超参数包括:
1. num_layers:Transformer模型的层数。
2. d_model:Transformer模型的隐藏层维数。
3. dff:Point-wise Feed Forward 全连接层的隐藏层维数。
4. num_heads:Multi-Head Attention 层的头数。
5. dropout_rate:Dropout层的丢弃率。
6. max_length:Transformer模型的最大序列长度。
7. target_vocab_size:目标语言的词汇表大小。
8. rate:学习率。
在TensorFlow中,我们可以使用tf.constant来定义这些超参数,代码如下:
```
num_layers = 6
d_model = 512
dff = 2048
num_heads = 8
dropout_rate = 0.1
max_length = 40
target_vocab_size = 8000
rate = 0.001
```
然后我们可以根据这些超参数构建Transformer模型。
相关问题
写一个用TensorFlow 实现的transfomer
实现 Transformer 模型需要考虑许多细节,因此我们将把它分为几个步骤:
1. 导入 TensorFlow 库。
2. 定义超参数,如词嵌入维数、多头注意力机制的头数、隐藏层维数等。
3. 实现位置编码。
4. 实现编码器和解码器的前馈传播。
5. 实现损失函数并定义优化器。
6. 训练模型并评估其效果。
下面是一个可以工作的代码示例:
```
import tensorflow as tf
# 定义超参数
num_layers = 6
d_model = 512
num_heads = 8
dff = 2048
input_vocab_size = 10000
target_vocab_size = 4000
dropout_rate = 0.1
# 定义位置编码
class PositionalEncoding(tf.keras.layers.Layer):
def __init__(self, position, d_model):
super(PositionalEncoding, self).__init__()
self.pos_encoding = self.positional_encoding(position, d_model)
def get_angles(self, position, i, d_model):
angles = 1 / tf.pow(10000, (2 * (i // 2)) / tf.cast(d_model, tf.float32))
return position * angles
def positional_encoding(self, position, d_model):
angle_rads = self.get_angles(
position=tf.range(position, dtype=tf.float32)[:, tf.newaxis],
i=tf.range(d_model, dtype=tf.float32)[tf.newaxis, :],
d_model=d_model)
# 将 sin 应用于奇数维,将 cos 应用于偶数维。
sines = tf.math.sin(angle_rads[:, 0::2])
cosines = tf.math.cos(angle_rads[:, 1::2])
pos_encoding = tf.concat([sines, cosines], axis=-1)
pos_encoding = pos_encoding[tf.newaxis, ...]
return tf.cast(pos_encoding, tf.float32
你能用python写一个多变量transfomer吗
当然可以。Transformer 是一种用于序列到序列转换的模型,它主要应用于自然语言处理任务。下面是一个基于 PyTorch 的多变量 Transformer 的简单实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class MultiVariableTransformer(nn.Module):
def __init__(self, input_dim, output_dim, n_layers=2, n_heads=8, hidden_dim=512, dropout=0.1):
super().__init__()
self.input_dim = input_dim
self.output_dim = output_dim
self.n_layers = n_layers
self.n_heads = n_heads
self.hidden_dim = hidden_dim
self.dropout = dropout
self.embedding = nn.Linear(input_dim, hidden_dim)
self.positional_encoding = PositionalEncoding(hidden_dim, dropout)
encoder_layer = nn.TransformerEncoderLayer(hidden_dim, n_heads, hidden_dim * 4, dropout)
self.encoder = nn.TransformerEncoder(encoder_layer, n_layers)
self.decoder = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
x = self.embedding(x)
x = self.positional_encoding(x)
x = self.encoder(x)
x = self.decoder(x.mean(dim=0))
return x
class PositionalEncoding(nn.Module):
def __init__(self, hidden_dim, dropout, max_len=5000):
super().__init__()
self.dropout = nn.Dropout(dropout)
pe = torch.zeros(max_len, hidden_dim)
position = torch.arange(0, max_len, dtype=torch.float).unsqueeze(1)
div_term = torch.exp(torch.arange(0, hidden_dim, 2).float() * (-math.log(10000.0) / hidden_dim))
pe[:, 0::2] = torch.sin(position * div_term)
pe[:, 1::2] = torch.cos(position * div_term)
pe = pe.unsqueeze(0).transpose(0, 1)
self.register_buffer('pe', pe)
def forward(self, x):
x = x + self.pe[:x.size(0), :]
return self.dropout(x)
```
此模型接受一个大小为 (seq_len, input_dim) 的输入张量,其中 seq_len 表示序列长度,input_dim 表示每个时间步的输入特征数。它返回一个大小为 (output_dim,) 的输出张量,其中 output_dim 表示输出特征数。该模型包含以下组件:
- `nn.Linear(input_dim, hidden_dim)`:输入嵌入层,将输入张量投影到 hidden_dim 维空间。
- `PositionalEncoding(hidden_dim, dropout)`:位置编码层,向输入张量添加位置编码。
- `nn.TransformerEncoder(encoder_layer, n_layers)`:编码器,将输入序列转换为表示。
- `nn.Linear(hidden_dim, output_dim)`:输出层,将表示投影到输出空间。
请注意,此代码中的 Transformer 模型仅用于演示目的。在实际应用中,您需要根据任务调整模型参数(如层数,头数和隐藏维度等)以获得更好的性能。
相关推荐
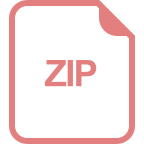
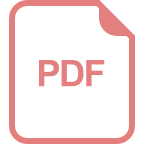
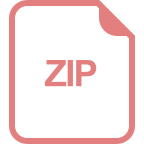












