mList = getClothesListbyUser(mSeason, mType) updateView(mList.size)第 一句是从数据库中查询数据,优化该代码,当数据库数据查询结束后再调用updateView(mList.size),用kotlin实现
时间: 2024-10-15 08:14:02 浏览: 20
在Kotlin中,你可以使用`withContext(Dispatchers.IO)`或者`launch(Dispatchers.IO)`来在IO线程上执行数据库查询,这样不会阻塞UI线程。以下是优化后的代码示例:
```kotlin
fun updateViewAfterDatabaseCall(season: String, type: String) {
GlobalScope.launch(Dispatchers.IO) {
val mList = getClothesListByUser(season, type)
// 当数据库查询结束并且获取到list后
withContext(Looper.getMainLooper()) {
updateView(mList?.size ?: 0) // 如果mList为空则传0,防止null安全问题
}
}
}
```
这里使用了`GlobalScope.launch`(推荐在非长期运行任务中使用,因为它不保存状态),它会启动一个新的Coroutine并在后台线程上执行。一旦查询完成,我们切换回主线程(`withContext(Looper.getMainLooper())`)来更新视图,确保UI流畅。
注意:`Dispatchers.IO`应该用于耗时操作,如网络请求或数据库查询,`Dispatchers.Main`用于UI相关的操作。
相关问题
解释这段代码: private void getdata() { List<Notice> noticeList = LitePal.findAll(Notice.class); if (noticeList != null && noticeList.size() > 0) { mlist.clear(); mlist.addAll(noticeList); allAdapter.notifyDataSetChanged(); } else { ToastUtils.showShort("公告暂时为空"); } top_recycleview.refreshComplete(); }
这是一个带有私有访问修饰符的方法,名为 `getdata()`。下面对代码逐行进行解释:
- 在第二行,使用 LitePal 数据库框架的 `findAll()` 方法从数据库中获取所有的 Notice 对象,并将它们存储在名为 `noticeList` 的 List 中。
- 在第三行,通过判断 `noticeList` 是否为空且元素个数大于 0 来进行条件判断。
- 如果条件满足,执行以下操作:
- 第四行,清空 `mlist` 中的元素。
- 第五行,将 `noticeList` 中的元素全部添加到 `mlist` 中。
- 第六行,通过调用 `allAdapter.notifyDataSetChanged()` 方法,通知与 `mlist` 相关联的适配器(`allAdapter`)数据源已发生改变,需要刷新显示。
- 如果条件不满足,执行以下操作:
- 第八行,通过 ToastUtils 工具类显示短时间的提示信息:"公告暂时为空"。
- 最后一行,调用 `top_recycleview.refreshComplete()` 方法,表示刷新操作已完成。
总结:该方法的作用是从数据库中获取 Notice 对象列表,并根据条件判断是否需要更新 `mlist` 数据源,并通过适配器来刷新界面显示。如果获取的列表为空,则会显示一个提示信息。最后,表示刷新操作已完成。
public View getView(int position, View convertView, ViewGroup parent) { View view=mLayoutInflater.inflate(R.layout.list_item,null); //取出数据赋值 Spinner tv_title = view.findViewById(R.id.tv_title); ArrayAdapter<String> adapter = new ArrayAdapter<>(context, android.R.layout.simple_spinner_item, mTitles); adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); tv_title.setAdapter(adapter); tv_title.setSelection(item.getTitleIndex()); costList item=mList.get(position); TextView tv_date=view.findViewById(R.id.tv_date); TextView tv_money=view.findViewById(R.id.tv_money); //绑定 tv_title.setText(null); tv_date.setText(mList.get(position).getDate()); tv_money.setText(mList.get(position).getMoney()); return view; }代码中显示context cannot resolve symbol context以及item cannot resolve symbol
这段代码中出现了两个错误:context cannot resolve symbol context和item cannot resolve symbol。这种情况通常是由于上下文(context)和变量(item)未定义或未导入所引起的。
要解决这个问题,你需要确保上下文和变量被正确定义和导入。上下文一般可以通过传递Activity或Fragment的上下文来解决(例如:getContext()或getActivity())。变量的定义和导入则需要检查是否有未声明的变量或未正确导入的库。
对于这段代码,你需要检查是否已经声明了context和item变量,并且它们的作用域是否正确。如果上下文是在Activity或Fragment中使用的,你可以使用getContext()或getActivity()方法来获取上下文。如果变量没有正确导入,你可以检查导入的库是否正确,或者手动导入缺少的库。
阅读全文
相关推荐
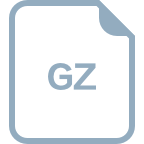
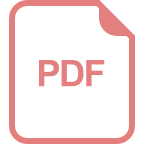
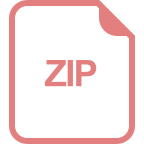












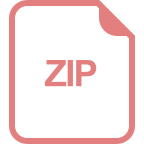
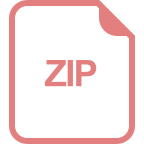