怎样在AccountController中实现自定义的身份验证流程?
时间: 2024-10-09 10:16:01 浏览: 27
在Spring MVC的`AccountController`中实现自定义身份验证流程通常涉及到以下几个步骤:
1. **创建认证服务** (AuthenticationService): 首先,你需要创建一个`AuthenticationService`接口,其中包含认证的方法,如`authenticate()`,它接收用户输入的凭据并进行验证。
```java
public interface AuthenticationService {
Authentication authenticate(UserCredentials credentials);
}
```
2. **实现认证服务**: 实现这个接口的某个类,比如`CustomAuthenticationService`,在这个类中编写具体的验证逻辑,可能包括检查数据库、校验密码哈希等。
```java
@Service
public class CustomAuthenticationService implements AuthenticationService {
@Autowired
private UserRepository userRepository;
@Override
public Authentication authenticate(UserCredentials credentials) {
User user = userRepository.findByUsername(credentials.getUsername());
if (user != null && passwordEncoder.matches(credentials.getPassword(), user.getPassword())) {
return new UsernamePasswordAuthenticationToken(user, "", user.getAuthorities());
}
throw new BadCredentialsException("Invalid credentials");
}
}
```
3. **配置`@ControllerAdvice`处理未授权**: 如果认证失败,可以创建一个全局异常处理器来捕获`AuthenticationException`并返回适当的响应。
```java
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(AuthenticationException.class)
public ResponseEntity<?> handleUnauthorized(Exception e) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
}
```
4. **在控制器中注入服务**并在需要的地方使用**: 在`AccountController`中,你需要注入`AuthenticationService`来进行身份验证。
```java
@RestController
@RequestMapping("/account")
public class AccountController {
private final AuthenticationService authenticationService;
@Autowired
public AccountController(AuthenticationService authenticationService) {
this.authenticationService = authenticationService;
}
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody UserCredentials credentials) {
Authentication authentication = authenticationService.authenticate(credentials);
// 进行后续操作,如设置会话...
}
}
```
阅读全文
相关推荐
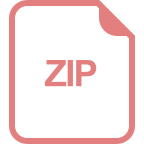
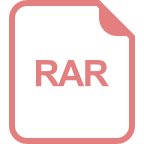
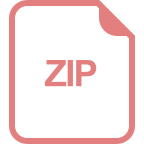
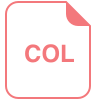
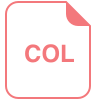
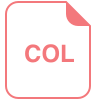
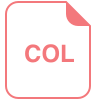
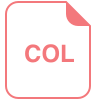
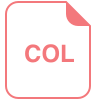
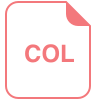
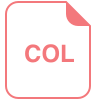

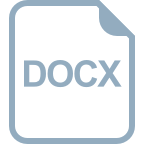
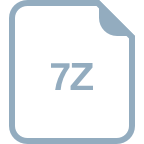