visual studio2017中使用ASP.NET Core MVC实现登录页面,包含手机、密码,使用注册页面登录
时间: 2024-09-12 15:12:10 浏览: 67
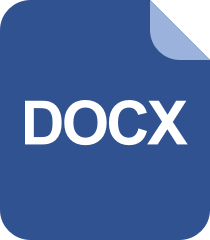
【ASP.NET编程知识】Visual Studio ASP.NET Core MVC入门教程第一篇.docx
在Visual Studio 2017中使用ASP.NET Core MVC实现登录页面通常涉及以下几个步骤:
1. **安装依赖**:首先确保已经安装了最新版本的.NET Core SDK和Visual Studio 2017。如果尚未安装,可以从官方Microsoft网站下载并安装。
2. **创建项目**:打开Visual Studio,选择“新建项目”,然后选择“ASP.NET Core Web Application”。选择MVC模板,并设置其他配置选项。
3. **身份验证设置**:在项目的Startup.cs文件中,启用Identity框架,这将提供基本的身份验证功能。添加`using Microsoft.AspNetCore.Identity;`,并在ConfigureServices和Configure中添加相关的中间件,如`app.UseAuthentication(); app.UseAuthorization();`
4. **模型类(Model)**:创建User模型类,用于存储用户信息,包括手机号和密码。可以继承`ApplicationUser`类并实现必要的属性和验证规则。
```csharp
public class ApplicationUser : IdentityUser<int>
{
public string PhoneNumber { get; set; }
}
```
5. **登录视图(View)设计**:在`Views/Account`文件夹下,新建一个Login.cshtml页面。这里需要使用`@model LoginModel`,其中`LoginModel`是你自定义的登录表单模型,包含手机号和密码字段。
6. **控制器(Controller)实现**:创建一个新的Controller,比如`AccountController`,重写`[HttpPost] [ValidateAntiForgeryToken] Login()`方法来处理登录请求。检查输入的数据,尝试从数据库中查找匹配的用户。
```csharp
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Login(LoginViewModel model)
{
if (ModelState.IsValid)
{
// 检查用户名和密码是否正确
var user = await _userManager.FindByEmailAsync(model.Email);
if (user != null && await _signInManager.PasswordCheckAsync(user, model.Password))
{
await _signInManager.SignInAsync(user, isPersistent: false);
return RedirectToAction("Index", "Home");
}
else
{
ModelState.AddModelError(string.Empty, "Invalid login credentials.");
}
}
// 如果有错误,返回到登录视图,显示错误消息
return View(model);
}
```
对于注册页面,你可以创建类似`Register.cshtml`的页面,以及处理注册的`Register()`方法,完成用户的注册流程后,也可以引导他们通过邮箱或手机验证码进行登录。
阅读全文
相关推荐
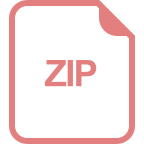
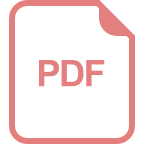

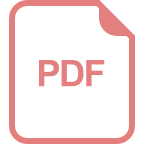
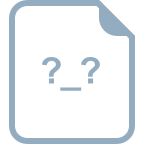
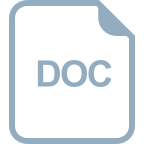
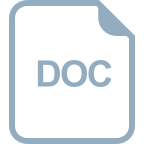
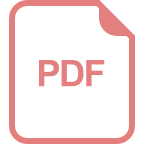
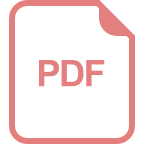
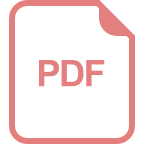
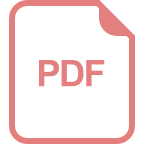
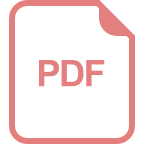
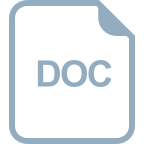
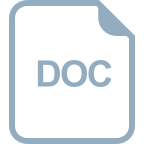

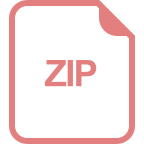
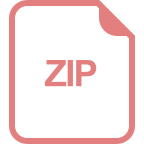
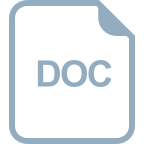