编程实现:栈的初始化、出栈、进栈操作,在main函数中设计菜单,测试进栈和出栈操作。
时间: 2023-05-17 10:06:57 浏览: 83
好的,以下是栈的初始化、出栈、进栈操作的示例代码:
```
#include <stdio.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int push(Stack *s, int x) {
if (s->top == MAXSIZE - 1) {
printf("Stack overflow\n");
return 0;
}
s->data[++(s->top)] = x;
return 1;
}
int pop(Stack *s, int *x) {
if (s->top == -1) {
printf("Stack underflow\n");
return 0;
}
*x = s->data[(s->top)--];
return 1;
}
int main() {
Stack s;
int choice, x;
initStack(&s);
while (1) {
printf("1. Push\n2. Pop\n3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter the element to be pushed: ");
scanf("%d", &x);
if (push(&s, x))
printf("%d pushed to stack\n", x);
break;
case 2:
if (pop(&s, &x))
printf("%d popped from stack\n", x);
break;
case 3:
return 0;
default:
printf("Invalid choice\n");
}
}
}
```
这段代码实现了栈的初始化、出栈、进栈操作,并在 main 函数中设计了菜单,可以测试进栈和出栈操作。
如果您有任何问题或需要进一步的解释,请随时问我。
相关推荐
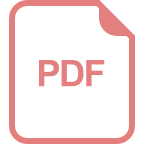














