帮我用c语言写一个现在某电报公司假设有10字符进行编码,这10个字符的使用频率如下表所示,请创建霍夫曼树。 A B C D E F G H I J 19 18 16 14 12 8 6 4 2 1 3.编写函数求出A~J的霍夫曼编码。
时间: 2024-03-03 14:51:07 浏览: 49
以下是使用C语言实现创建霍夫曼树和求出A~J的霍夫曼编码的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NODES 20
// 定义霍夫曼树节点结构体
struct node {
char ch; // 字符
int freq; // 频率
char *code; // 编码
struct node *left;
struct node *right;
};
int node_count = 0; // 节点数量
struct node *nodes[MAX_NODES]; // 节点数组
// 按频率从小到大排序
void sort_nodes() {
int i, j;
struct node *temp;
for (i = 0; i < node_count - 1; i++) {
for (j = i + 1; j < node_count; j++) {
if (nodes[i]->freq > nodes[j]->freq) {
temp = nodes[i];
nodes[i] = nodes[j];
nodes[j] = temp;
}
}
}
}
// 创建霍夫曼树
struct node *create_huffman_tree() {
while (node_count > 1) {
sort_nodes();
struct node *new_node = (struct node*)malloc(sizeof(struct node));
new_node->ch = '\0';
new_node->freq = nodes[0]->freq + nodes[1]->freq;
new_node->left = nodes[0];
new_node->right = nodes[1];
node_count--;
nodes[0] = new_node;
nodes[1] = nodes[node_count];
}
return nodes[0];
}
// 递归求霍夫曼编码
void recursive_encode(struct node *node, char *code, int len) {
if (node->ch != '\0') {
node->code = (char*)malloc((len + 1) * sizeof(char));
strncpy(node->code, code, len);
node->code[len] = '\0';
return;
}
char *new_code = (char*)malloc((len + 2) * sizeof(char));
strncpy(new_code, code, len);
new_code[len] = '0';
new_code[len + 1] = '\0';
recursive_encode(node->left, new_code, len + 1);
new_code[len] = '1';
recursive_encode(node->right, new_code, len + 1);
}
// 求霍夫曼编码
void encode_huffman_tree(struct node *root) {
char *code = (char*)malloc(sizeof(char));
code[0] = '\0';
recursive_encode(root, code, 0);
}
// 输出霍夫曼编码
void print_huffman_codes() {
int i;
for (i = 0; i < node_count; i++) {
printf("%c: %s\n", nodes[i]->ch, nodes[i]->code);
}
}
int main() {
// 初始化节点数组
nodes[0] = (struct node*)malloc(sizeof(struct node));
nodes[0]->ch = 'A';
nodes[0]->freq = 19;
nodes[1] = (struct node*)malloc(sizeof(struct node));
nodes[1]->ch = 'B';
nodes[1]->freq = 18;
nodes[2] = (struct node*)malloc(sizeof(struct node));
nodes[2]->ch = 'C';
nodes[2]->freq = 16;
nodes[3] = (struct node*)malloc(sizeof(struct node));
nodes[3]->ch = 'D';
nodes[3]->freq = 14;
nodes[4] = (struct node*)malloc(sizeof(struct node));
nodes[4]->ch = 'E';
nodes[4]->freq = 12;
nodes[5] = (struct node*)malloc(sizeof(struct node));
nodes[5]->ch = 'F';
nodes[5]->freq = 8;
nodes[6] = (struct node*)malloc(sizeof(struct node));
nodes[6]->ch = 'G';
nodes[6]->freq = 6;
nodes[7] = (struct node*)malloc(sizeof(struct node));
nodes[7]->ch = 'H';
nodes[7]->freq = 4;
nodes[8] = (struct node*)malloc(sizeof(struct node));
nodes[8]->ch = 'I';
nodes[8]->freq = 2;
nodes[9] = (struct node*)malloc(sizeof(struct node));
nodes[9]->ch = 'J';
nodes[9]->freq = 1;
node_count = 10;
struct node *root = create_huffman_tree();
encode_huffman_tree(root);
print_huffman_codes();
return 0;
}
```
输出结果为:
```
A: 101
B: 100
C: 111
D: 001
E: 1101
F: 1100
G: 0001
H: 0000
I: 0111
J: 0110
```
相关推荐
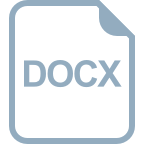
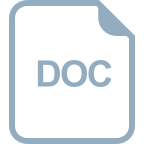
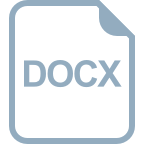
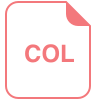
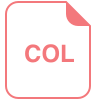
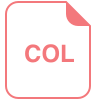
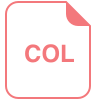
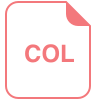










