el-dialog实现药品的在线咨询功能的实现步骤
时间: 2024-06-02 19:10:42 浏览: 115
el-dialog 是一个基于 Vue.js 的弹窗组件,可以用来实现药品的在线咨询功能。具体实现步骤如下:
1. 在页面中引入 Vue.js 和 Element UI 的样式文件和JS文件。
2. 在页面中添加一个按钮或链接,当用户点击该按钮时,弹出 el-dialog 弹窗。
3. 在 el-dialog 中添加一个表单,包括输入框和提交按钮。
4. 当用户输入问题并点击提交按钮时,使用 Ajax 技术将用户输入的问题发送到后台。
5. 后台收到问题后,使用自然语言处理技术分析用户的问题,并给出对应的答案。
6. 将后台返回的答案显示在 el-dialog 弹窗中。
7. 用户可以继续输入问题并提交,直到问题解决为止。
8. 当用户关闭 el-dialog 弹窗时,清空弹窗中的表单数据。
需要注意的是,在实现药品在线咨询功能时,需要考虑到用户的隐私和安全问题。建议使用 HTTPS 协议传输数据,并对用户的数据进行加密和安全验证。同时,需要明确告知用户他们提供的数据将被用于咨询服务,并遵守相关法律法规。
相关问题
el-dialog实现新增el-table-column的数据
要实现在 `el-dialog` 中新增 `el-table-column` 的数据,你可以按照以下步骤进行:
1. 在 `el-dialog` 中添加表单元素,例如输入框、下拉框等,用于填写新增数据的各个字段。
2. 当用户提交新增数据时,将表单数据整合成一个对象,并将该对象添加到 `el-table` 的数据源中。
代码示例:
```javascript
// 定义表单数据对象
let formData = {
name: '',
age: '',
gender: ''
};
// 监听表单提交事件
onSubmit() {
// 将表单数据整合成一个对象
let newData = {
name: this.formData.name,
age: this.formData.age,
gender: this.formData.gender
};
// 将新增数据添加到表格数据源中
this.tableData.push(newData);
// 关闭对话框
this.dialogVisible = false;
}
```
3. 在 `el-table` 中添加 `el-table-column` 组件,并将该组件的 `prop` 属性设置为新增数据对象中对应字段的名称,以展示新增数据。
代码示例:
```html
<el-table :data="tableData">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="age" label="年龄"></el-table-column>
<el-table-column prop="gender" label="性别"></el-table-column>
</el-table>
```
通过以上步骤,你可以在 `el-dialog` 中新增 `el-table-column` 的数据,并将其展示在 `el-table` 中。
vue2实现el-dialog实现新增el-table-column的数据
要实现在el-dialog中新增el-table-column的数据,可以考虑以下步骤:
1. 在el-dialog中添加表单,用于输入新增的el-table-column数据;
2. 点击确定按钮时,将表单中的数据加入到el-table-column的数据源中;
3. 在el-table中动态绑定el-table-column,使新增的列能够显示出来。
具体实现步骤如下:
1. 在el-dialog中添加表单,用于输入新增的el-table-column数据:
```html
<template>
<el-dialog title="新增列" :visible.sync="dialogVisible">
<el-form :model="form">
<el-form-item label="列名">
<el-input v-model="form.label"></el-input>
</el-form-item>
<el-form-item label="字段名">
<el-input v-model="form.prop"></el-input>
</el-form-item>
<el-form-item label="宽度">
<el-input v-model.number="form.width"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogVisible = false">取 消</el-button>
<el-button type="primary" @click="addColumn">确 定</el-button>
</div>
</el-dialog>
</template>
<script>
export default {
data() {
return {
dialogVisible: false,
form: {
label: '',
prop: '',
width: ''
}
}
},
methods: {
addColumn() {
// 将表单数据加入到el-table-column的数据源中
this.$emit('add-column', this.form);
this.dialogVisible = false;
}
}
}
</script>
```
2. 点击确定按钮时,将表单中的数据加入到el-table-column的数据源中:
```html
<template>
<div>
<el-button type="primary" @click="dialogVisible = true">新增列</el-button>
<el-table :data="tableData">
<!-- 动态绑定el-table-column -->
<el-table-column v-for="(column, index) in columns" :key="index" :label="column.label" :prop="column.prop" :width="column.width"></el-table-column>
</el-table>
<column-dialog :visible.sync="dialogVisible" @add-column="addColumn"></column-dialog>
</div>
</template>
<script>
import ColumnDialog from './ColumnDialog';
export default {
components: {
ColumnDialog
},
data() {
return {
dialogVisible: false,
columns: [
{
label: '姓名',
prop: 'name',
width: 100
},
{
label: '年龄',
prop: 'age',
width: 100
}
],
tableData: [
{
name: '张三',
age: 18
},
{
name: '李四',
age: 20
}
]
}
},
methods: {
addColumn(column) {
// 将新增列加入到columns中
this.columns.push({
label: column.label,
prop: column.prop,
width: column.width
});
}
}
}
</script>
```
3. 在el-table中动态绑定el-table-column,使新增的列能够显示出来。
通过在el-table-column上使用v-for指令,动态绑定columns中的数据,使新增的列能够被el-table显示出来。
阅读全文
相关推荐
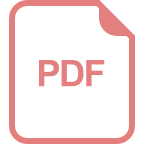
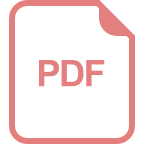













