WebRTC的RTCPeerConnection 怎么把媒体流发送出去
时间: 2024-11-16 17:19:34 浏览: 9
WebRTC 的 RTCPeerConnection(Real-time Transport Control Protocol Peer Connection)是用于实时通信的核心组件,它提供了点对点的实时音视频通信能力。如果你想把媒体流(如音频和视频)从一个设备发送到另一个设备,通常需要以下步骤:
1. **初始化 PeerConnection**:
首先,创建一个新的 `RTCPeerConnection` 实例,并可能加入到某个已存在的 STUN 或 TURN 服务器列表中,以便解决 NAT 穿越问题。
```javascript
async function createPeerConnection() {
const pc = new RTCPeerConnection({
iceServers: [{ urls: 'stun:stun.l.google.com:19302' }],
});
await pc.readyToNegotiate();
return pc;
}
```
2. **添加媒体流(offerer)**:
当你想发起连接时,作为 offerer(主动方),你可以使用 `pc.createOffer()` 创建一个offer,这包含了你的设备愿意提供和接受的内容。
```javascript
await pc.createOffer()
.then(offer => pc.setLocalDescription(offer))
.then(description => sendDescriptionToOtherDevice(description));
```
3. **发送描述(offer)**:
将 offer 发送给对方(answerer),例如通过 signaling server,将 SDP(Session Description Protocol)字符串传递过去。
```javascript
function sendDescriptionToOtherDevice(description) {
fetch('/set-answer', {
method: 'POST',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify({ type: 'offer', sdp: description.sdp }),
}).then(response => response.json());
}
```
4. **接收回应(answer)**:
对方接收到offer后,会在他们的端点创建 answer 并返回给你。你需要解析并设置到本地 peerconnection。
```javascript
fetch('/get-answer')
.then(response => response.json())
.then(answer => pc.setRemoteDescription(new RTCSessionDescription(answer)));
```
5. **添加接收者的流**:
使用 `pc.addStream(otherUserStream)` 添加远程用户提供的媒体流,这样你就可以在本地播放了。
6. **创建 ICE 和 DTLS 通道**:
`RTCPeerConnection` 自动处理 ICE (Interactive Connectivity Establishment) 和 DTLS (Datagram Transport Layer Security) 的连接过程,它们会自动尝试找到稳定的网络路径。
7. **打开音频和视频轨道**:
调整音频和视频的 `track` 到渲染器(如 `<video>` 元素)上,显示媒体流。
```javascript
pc.getAudioTracks().forEach(track => video.srcObject = track);
```
阅读全文
相关推荐
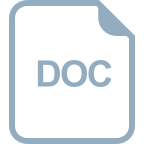
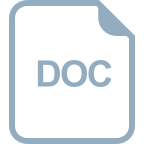
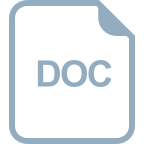
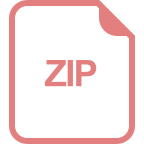
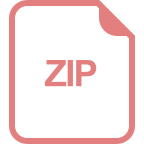
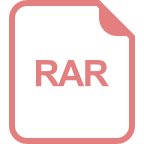
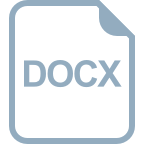
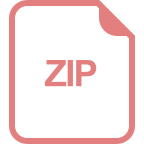
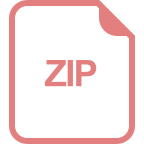
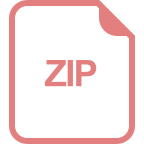
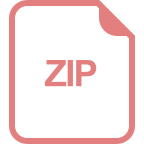
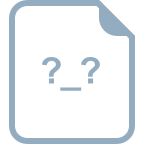
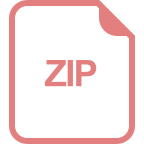
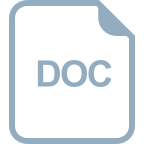
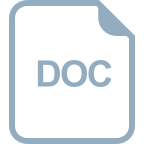
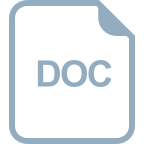
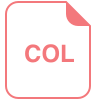