def get_queryset(self, request): qs = warehousePeople.objects.all() if 'name' in request.GET and request.GET['name'] != 'none': # 条件查询 qs = qs.filter(name=request.GET['name']) qs1 = qs.none() if 'subjectMatter' in self.list_display: self.list_display.remove('subjectMatter') if 'apply_prove' not in self.list_display: self.list_display.append('apply_prove') for obj in qs: if qs1.filter(month=obj.month, warehouse=obj.warehouse.id).exists(): break else: infoLi = qs.filter(month=obj.month, warehouse=obj.warehouse.id) first_obj = infoLi.first() infoLiOne = qs.filter(pk=first_obj.pk) qs1 |= infoLiOne else: if 'subjectMatter' not in self.list_display: self.list_display.append('subjectMatter') if "apply_prove" in self.list_display: self.list_display.remove('apply_prove') if 'name' in request.GET and request.GET['name'] != 'none': return qs1 else: return qs 代码中如何使用annotate方法动态的为qs1添加动态字段apply_prove?
时间: 2024-02-10 13:08:17 浏览: 44
可以使用annotate方法来为qs1添加动态字段apply_prove,具体代码如下:
```
from django.db.models import Case, When, IntegerField
qs1 = qs.none().annotate(
apply_prove=Case(
When(warehouse__isnull=True, then=0),
When(month__isnull=True, then=0),
default=1,
output_field=IntegerField(),
)
)
```
这段代码中,我们使用了annotate方法来为qs1添加一个名为apply_prove的动态字段。annotate方法可以接收多个参数,每个参数都是一个表达式,用来为查询集中每个对象添加一个字段。在这个例子中,我们使用了Case表达式来根据warehouse和month是否为空来计算apply_prove的值。具体来说,当warehouse或month为空时,apply_prove的值就为0,否则就为1。最后,我们指定了这个动态字段的输出类型为IntegerField。
相关问题
def get_queryset(self, request): qs = super().get_queryset(request) result = [] if 'name' in request.GET and request.GET['name'] != 'none': # 条件查询 qs = warehousePeople.objects.filter(name=request.GET['name']) if 'subjectMatter' in self.list_display: self.list_display.remove('subjectMatter') if 'apply_prove' not in self.list_display: self.list_display.append('apply_prove') qs = qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct() for item in qs: warehouse = warehouseInformation.objects.get(id=item['warehouse']) obj = warehousePeople(month=item['month'], warehouse=warehouse) obj.apply_prove = item['apply_prove'] result.append(obj) 怎么将result转换为QuerySet,并且保留result列表中自定义字段apply_prove 代码应该怎么修改
要将`result`转换为`QuerySet`并保留自定义字段`apply_prove`,可以使用`QuerySet`的`values()`和`annotate()`方法来实现。下面是修改后的代码:
```python
from django.db.models import Count
def get_queryset(self, request):
qs = super().get_queryset(request)
result = []
if 'name' in request.GET and request.GET['name'] != 'none':
# 条件查询
qs = warehousePeople.objects.filter(name=request.GET['name'])
if 'subjectMatter' in self.list_display:
self.list_display.remove('subjectMatter')
if 'apply_prove' not in self.list_display:
self.list_display.append('apply_prove')
qs = qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct()
for item in qs:
warehouse = warehouseInformation.objects.get(id=item['warehouse'])
obj = warehousePeople(month=item['month'], warehouse=warehouse)
obj.apply_prove = item['apply_prove']
result.append(obj)
# 将result转换为QuerySet并保留自定义字段apply_prove
qs = warehousePeople.objects.none()
for obj in result:
qs |= warehousePeople.objects.filter(month=obj.month, warehouse=obj.warehouse).values(*self.list_display).annotate(apply_prove=obj.apply_prove)
return qs
```
这里使用了`qs.values('month', 'warehouse').annotate(apply_prove=Count('id')).distinct()`来计算每个月、每个仓库的`apply_prove`值,并将结果存储在`item['apply_prove']`中。然后,将每个`item`转换为`warehousePeople`对象,并将`apply_prove`值存储在`obj.apply_prove`中。最后,使用`|=`操作符将每个`obj`对应的`QuerySet`合并到`qs`中,并返回`qs`。
from django.views.generic import ListView class RankingList(ListView): context_object_name = 'dynamics' template_name = 'ranking.html' def get_queryset(self): t = self.request.GET.get('type', '') if t: dynamics = Dynamic.objects.select_related('video').filter(video__label=t).order_by('-plays').all()[:10] else: dynamics = Dynamic.objects.select_related('video').order_by('-plays').all()[:10] return dynamics def get_context_data(self, **kwargs): context = super().get_context_data(**kwargs) context['searchs'] = Dynamic.objects.select_related('video').order_by('-search').all()[:4] context['labels'] = Label.objects.all() return context
这段代码定义了一个名为 RankingList 的类视图,继承自 Django 中的 ListView。其中,context_object_name 属性指定了返回给模板的查询结果的上下文变量名为 dynamics,template_name 属性指定了使用的模板文件为 ranking.html。
get_queryset 方法是 ListView 中用于获取查询结果集的方法,这里按照与 rankingView 中相同的逻辑进行查询,并返回查询结果。
get_context_data 方法是用于获取模板上下文数据的方法,这里通过调用父类的方法获取上下文数据,然后再添加查询结果 searchs 和 labels,并最终返回整个上下文数据字典。
相关推荐
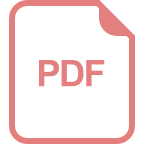
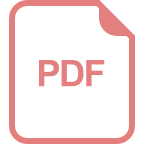
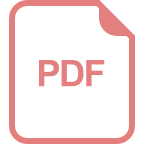












