通讯录排序:通信录的结构记录包括姓名、生日、电话号码,其中生日又包括年、月、日这三项。定义一个嵌套的结构类型,输入口个联系人的信息,再接地们的年給以大郵小的顺序依次输出其信息。试编写相应程序。
时间: 2023-12-06 15:44:13 浏览: 96
以下是使用C语言实现的通讯录排序程序:
```c
#include <stdio.h>
struct date {
int year;
int month;
int day;
};
struct contact {
char name[20];
struct date birthday;
char phone[12];
};
int compare(struct date d1, struct date d2) {
if (d1.year != d2.year) {
return d1.year - d2.year;
} else if (d1.month != d2.month) {
return d1.month - d2.month;
} else {
return d1.day - d2.day;
}
}
void sort(struct contact contacts[], int n) {
int i, j;
struct contact temp;
for (i = 0; i < n - 1; i++) {
for (j = i + 1; j < n; j++) {
if (compare(contacts[i].birthday, contacts[j].birthday) > 0) {
temp = contacts[i];
contacts[i] = contacts[j];
contacts[j] = temp;
}
}
}
}
int main() {
int n, i;
printf("Enter the number of contacts: ");
scanf("%d", &n);
struct contact contacts[n];
for (i = 0; i < n; i++) {
printf("Enter the name, birthday (year month day), and phone number of contact %d: ", i + 1);
scanf("%s %d %d %d %s", contacts[i].name, &contacts[i].birthday.year, &contacts[i].birthday.month, &contacts[i].birthday.day, contacts[i].phone);
}
sort(contacts, n);
printf("Contacts sorted by birthday:\n");
for (i = 0; i < n; i++) {
printf("%s\t%d-%02d-%02d\t%s\n", contacts[i].name, contacts[i].birthday.year, contacts[i].birthday.month, contacts[i].birthday.day, contacts[i].phone);
}
return 0;
}
```
程序中定义了两个结构体类型,一个是`date`,用来表示生日日期,另一个是`contact`,用来表示联系人信息。在`compare`函数中,将两个日期进行比较,返回值为负数表示第一个日期比第二个日期早,返回值为正数表示第一个日期比第二个日期晚,返回值为0表示两个日期相同。在`sort`函数中,使用冒泡排序算法将联系人信息按照生日日期从早到晚排序。在`main`函数中,先读入联系人信息,然后调用`sort`函数进行排序,并输出排序后的联系人信息。
注意,程序中没有对输入的生日日期进行合法性检查,实际应用中需要进行相应的检查。
相关推荐
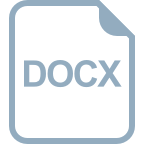
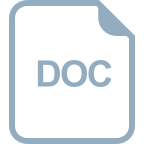
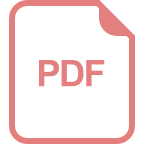












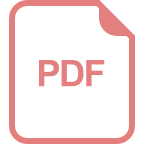
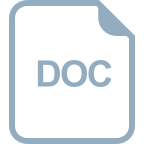
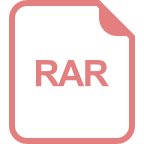
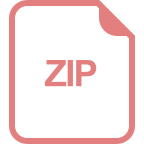