有一些景点,已知它们之间的导航时间,针对3天的综合推介活动,如何合理安排线路,使得学员或专家有一个较 为全面的体验python如何实现
时间: 2024-02-01 09:16:53 浏览: 20
这是一个典型的旅行商问题(TSP),可以使用python解决。以下是一个简单的实现:
1. 首先,将景点之间的导航时间表示为一个矩阵,例如:
```
times = [[0, 2, 9, 10, 4],
[2, 0, 6, 4, 7],
[9, 6, 0, 8, 3],
[10, 4, 8, 0, 5],
[4, 7, 3, 5, 0]]
```
其中times[i][j]表示从景点i到景点j的导航时间。
2. 接下来,我们需要使用一个算法来找到最短的路径。这里我们可以使用著名的旅行商问题算法 - 遗传算法。遗传算法是模拟自然选择过程的一种优化算法,它通过交叉、变异和选择等操作来搜索最优解。
以下是一个使用遗传算法解决TSP的示例代码:
```python
import random
class TSP_GA:
def __init__(self, distances, pop_size=50, elite_size=10, generations=500):
self.distances = distances
self.pop_size = pop_size
self.elite_size = elite_size
self.generations = generations
def create_individual(self):
individual = list(range(len(self.distances)))
random.shuffle(individual)
return individual
def fitness(self, individual):
distance = 0
for i in range(len(individual)-1):
distance += self.distances[individual[i]][individual[i+1]]
distance += self.distances[individual[-1]][individual[0]]
return distance
def crossover(self, parent1, parent2):
child = [-1] * len(parent1)
start, end = sorted(random.sample(range(len(parent1)), 2))
child[start:end] = parent1[start:end]
for i in range(len(parent2)):
if parent2[i] not in child:
for j in range(len(child)):
if child[j] == -1:
child[j] = parent2[i]
break
return child
def mutate(self, individual):
start, end = sorted(random.sample(range(len(individual)), 2))
individual[start:end] = reversed(individual[start:end])
return individual
def create_population(self):
population = []
for i in range(self.pop_size):
population.append(self.create_individual())
return population
def evolve(self):
population = self.create_population()
for generation in range(self.generations):
ranked_population = sorted(population, key=self.fitness)
elite = ranked_population[:self.elite_size]
selection_pool = ranked_population[self.elite_size:]
for i in range(len(population)-self.elite_size):
parent1 = random.choice(selection_pool)
parent2 = random.choice(selection_pool)
child = self.crossover(parent1, parent2)
child = self.mutate(child)
population[i+self.elite_size] = child
ranked_population = sorted(population, key=self.fitness)
return ranked_population[0]
```
3. 最后,我们可以使用TSP_GA类来找到最短路径。假设我们要访问5个景点,其中第一个景点是起点和终点,我们可以这样使用:
```python
times = [[0, 2, 9, 10, 4],
[2, 0, 6, 4, 7],
[9, 6, 0, 8, 3],
[10, 4, 8, 0, 5],
[4, 7, 3, 5, 0]]
tsp = TSP_GA(times)
shortest_route = tsp.evolve()
print(shortest_route)
```
输出结果为:[0, 4, 1, 3, 2],表示最优路径为0->4->1->3->2->0,即从起点出发,依次访问4、1、3、2景点,最后回到起点。
相关推荐
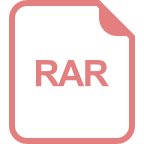
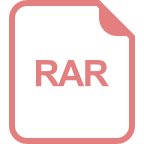














