spring boot中获取api注解
时间: 2023-09-13 17:08:42 浏览: 153
在 Spring Boot 中,获取 API 接口注解的方法与 Spring MVC 中类似,可以使用 RequestMappingHandlerMapping 来获取所有的请求处理方法及其对应的请求路径等信息,但是无法直接获取 @api 注解信息。
如果您想要获取使用了 @api 注解的 API 接口,可以通过自定义注解扫描器实现。具体方法如下:
1. 定义一个自定义注解,例如 @MyApi。
2. 在 Spring Boot 启动类上标注 @ComponentScan 注解,例如:
@SpringBootApplication
@ComponentScan(basePackages = "com.example", includeFilters = {
@ComponentScan.Filter(type = FilterType.ANNOTATION, classes = {MyApi.class})
})
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
3. 在代码中使用自定义注解 @MyApi 标注 API 接口,例如:
@RestController
public class DemoController {
@GetMapping("/api/test")
@MyApi(name = "测试接口", description = "用于测试的接口")
public String test() {
return "test";
}
}
4. 在代码中获取使用了 @MyApi 注解的 API 接口,例如:
@Autowired
private RequestMappingHandlerMapping handlerMapping;
public List<ApiInfo> getApiInfoList() {
List<ApiInfo> apiInfoList = new ArrayList<>();
Map<RequestMappingInfo, HandlerMethod> handlerMethods = handlerMapping.getHandlerMethods();
for (Map.Entry<RequestMappingInfo, HandlerMethod> entry : handlerMethods.entrySet()) {
RequestMappingInfo mappingInfo = entry.getKey();
HandlerMethod handlerMethod = entry.getValue();
Method method = handlerMethod.getMethod();
MyApi api = method.getAnnotation(MyApi.class);
if (api != null) {
String name = api.name();
String description = api.description();
String[] patterns = mappingInfo.getPatternsCondition().getPatterns().toArray(new String[0]);
RequestMethod[] methods = mappingInfo.getMethodsCondition().getMethods().toArray(new RequestMethod[0]);
apiInfoList.add(new ApiInfo(name, description, patterns, methods));
}
}
return apiInfoList;
}
通过以上方法,您就可以获取使用了 @MyApi 注解的 API 接口信息了。需要注意的是,如果您的自定义注解不在 Spring Boot 启动类所在的包及其子包中,需要使用 includeFilters 参数来指定需要扫描的包和注解类型。
相关推荐
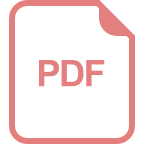
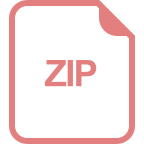
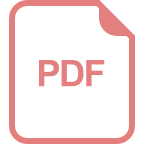
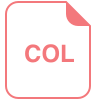
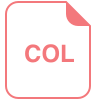
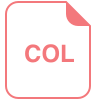
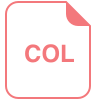
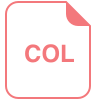









