VxWorks检查文件是否已创建的函数
时间: 2024-06-11 11:04:56 浏览: 112
VxWorks提供了许多函数来检查文件是否已创建,以下是其中几个常用的函数:
1. access()函数:检查文件是否存在。
```
#include <unistd.h>
int access(const char *path, int amode);
```
参数说明:
- `path`:文件路径。
- `amode`:访问模式,可以是`F_OK`(检查文件是否存在)、`R_OK`(检查文件是否可读)、`W_OK`(检查文件是否可写)和`X_OK`(检查文件是否可执行)。
返回值:
- 若文件存在且有相应权限,则返回0。
- 若文件不存在或无相应权限,则返回-1,并设置errno。
示例代码:
```c
if (access("/path/to/file", F_OK) == 0) {
printf("File exists.\n");
} else {
printf("File does not exist.\n");
}
```
2. stat()函数:获取文件信息。
```
#include <sys/stat.h>
int stat(const char *path, struct stat *buf);
```
参数说明:
- `path`:文件路径。
- `buf`:存储文件信息的结构体。
返回值:
- 若成功,返回0。
- 若失败,返回-1,并设置errno。
示例代码:
```c
struct stat st;
if (stat("/path/to/file", &st) == 0) {
printf("File size is %ld bytes.\n", st.st_size);
} else {
printf("Failed to get file information.\n");
}
```
3. open()函数:打开文件。
```
#include <fcntl.h>
int open(const char *path, int flags, ...);
```
参数说明:
- `path`:文件路径。
- `flags`:打开模式,可以是`O_RDONLY`(只读)、`O_WRONLY`(只写)和`O_RDWR`(读写)等。
- ...:可选参数,用于指定文件的权限,例如`S_IRUSR`(用户可读)和`S_IWUSR`(用户可写)等。
返回值:
- 若成功,返回文件描述符。
- 若失败,返回-1,并设置errno。
示例代码:
```c
int fd = open("/path/to/file", O_RDONLY);
if (fd >= 0) {
printf("File opened successfully.\n");
close(fd);
} else {
printf("Failed to open file.\n");
}
```
以上是常用的几个函数,还有其他函数如access()、chdir()、chroot()等也可以用于检查文件是否已创建。
阅读全文
相关推荐


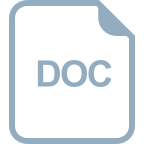





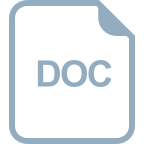



