c# 实现whoami /priv命令 不使用Process类 完整代码
时间: 2024-03-11 10:50:28 浏览: 19
以下是使用 C# 实现 whoami /priv 命令,不使用 Process 类的完整代码:
```csharp
using System;
using System.Runtime.InteropServices;
namespace WhoAmI
{
class Program
{
[DllImport("advapi32.dll", SetLastError = true)]
static extern bool OpenProcessToken(IntPtr ProcessHandle, uint DesiredAccess, out IntPtr TokenHandle);
[DllImport("advapi32.dll", SetLastError = true)]
static extern bool LookupPrivilegeName(string lpSystemName, IntPtr lpLuid, System.Text.StringBuilder lpName, ref int cchName);
[DllImport("advapi32.dll", SetLastError = true)]
static extern bool LookupPrivilegeValue(string lpSystemName, string lpName, out LUID lpLuid);
[DllImport("advapi32.dll", SetLastError = true)]
static extern bool GetTokenInformation(IntPtr TokenHandle, TOKEN_INFORMATION_CLASS TokenInformationClass, IntPtr TokenInformation, uint TokenInformationLength, out uint ReturnLength);
[DllImport("kernel32.dll", SetLastError = true)]
static extern IntPtr GetCurrentProcess();
static void Main(string[] args)
{
IntPtr tokenHandle;
if (!OpenProcessToken(GetCurrentProcess(), 0x8 /*TOKEN_QUERY*/, out tokenHandle))
{
Console.WriteLine("Failed to get token handle");
return;
}
uint returnLength;
if (!GetTokenInformation(tokenHandle, TOKEN_INFORMATION_CLASS.TokenPrivileges, IntPtr.Zero, 0, out returnLength))
{
int lastError = Marshal.GetLastWin32Error();
if (lastError != ERROR_INSUFFICIENT_BUFFER)
{
Console.WriteLine("Failed to get token information");
return;
}
}
IntPtr tokenInformation = Marshal.AllocHGlobal((int)returnLength);
if (!GetTokenInformation(tokenHandle, TOKEN_INFORMATION_CLASS.TokenPrivileges, tokenInformation, returnLength, out returnLength))
{
Console.WriteLine("Failed to get token information");
return;
}
TOKEN_PRIVILEGES privileges = (TOKEN_PRIVILEGES)Marshal.PtrToStructure(tokenInformation, typeof(TOKEN_PRIVILEGES));
Console.WriteLine("User privileges:");
for (int i = 0; i < privileges.PrivilegeCount; i++)
{
LUID_AND_ATTRIBUTES luidAndAttributes = privileges.Privileges[i];
int nameLength = 0;
LookupPrivilegeName(null, luidAndAttributes.Luid, null, ref nameLength);
System.Text.StringBuilder nameBuilder = new System.Text.StringBuilder(nameLength);
if (LookupPrivilegeName(null, luidAndAttributes.Luid, nameBuilder, ref nameLength))
{
Console.WriteLine("- " + nameBuilder.ToString());
}
else
{
Console.WriteLine("- Unknown privilege");
}
}
Marshal.FreeHGlobal(tokenInformation);
}
private const int ERROR_INSUFFICIENT_BUFFER = 122;
private enum TOKEN_INFORMATION_CLASS
{
TokenUser = 1,
TokenGroups,
TokenPrivileges,
TokenOwner,
TokenPrimaryGroup,
TokenDefaultDacl,
TokenSource,
TokenType,
TokenImpersonationLevel,
TokenStatistics,
TokenRestrictedSids,
TokenSessionId,
TokenGroupsAndPrivileges,
TokenSessionReference,
TokenSandBoxInert,
TokenAuditPolicy,
TokenOrigin,
TokenElevationType,
TokenLinkedToken,
TokenElevation,
TokenHasRestrictions,
TokenAccessInformation,
TokenVirtualizationAllowed,
TokenVirtualizationEnabled,
TokenIntegrityLevel,
TokenUIAccess,
TokenMandatoryPolicy,
TokenLogonSid,
MaxTokenInfoClass
}
[StructLayout(LayoutKind.Sequential)]
private struct LUID
{
public uint LowPart;
public int HighPart;
}
[StructLayout(LayoutKind.Sequential)]
private struct LUID_AND_ATTRIBUTES
{
public LUID Luid;
public uint Attributes;
}
[StructLayout(LayoutKind.Sequential)]
private struct TOKEN_PRIVILEGES
{
public int PrivilegeCount;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 1)]
public LUID_AND_ATTRIBUTES[] Privileges;
}
}
}
```
该程序首先调用 OpenProcessToken 函数打开当前进程的访问令牌并获取其句柄,然后调用 GetTokenInformation 函数获取令牌的特权信息。最后,程序将特权信息打印到控制台。注意,此代码仅适用于 Windows 操作系统。
相关推荐
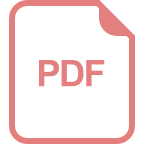
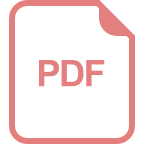














