c# 实现whoami /priv命令 使用LookupPrivilegeName 获取特权信息 完整代码
时间: 2024-03-11 18:50:06 浏览: 18
好的,以下是 C# 实现 whoami /priv 命令并使用 LookupPrivilegeName 获取特权信息的完整代码:
```
using System;
using System.Runtime.InteropServices;
class Program
{
private const int SE_PRIVILEGE_ENABLED = 0x00000002;
private const int TOKEN_QUERY = 0x00000008;
private const int TOKEN_ADJUST_PRIVILEGES = 0x00000020;
private const int MAX_NAME_LENGTH = 1024;
[StructLayout(LayoutKind.Sequential)]
public struct LUID
{
public uint LowPart;
public int HighPart;
}
[StructLayout(LayoutKind.Sequential)]
public struct LUID_AND_ATTRIBUTES
{
public LUID Luid;
public uint Attributes;
}
[StructLayout(LayoutKind.Sequential)]
public struct TOKEN_PRIVILEGES
{
public uint PrivilegeCount;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 1)]
public LUID_AND_ATTRIBUTES[] Privileges;
}
[DllImport("advapi32.dll", SetLastError = true)]
[return: MarshalAs(UnmanagedType.Bool)]
public static extern bool OpenProcessToken(IntPtr ProcessHandle, uint DesiredAccess, out IntPtr TokenHandle);
[DllImport("advapi32.dll", SetLastError = true)]
[return: MarshalAs(UnmanagedType.Bool)]
public static extern bool LookupPrivilegeName(string lpSystemName, IntPtr lpLuid, System.Text.StringBuilder lpName, ref int cchName);
[DllImport("advapi32.dll", SetLastError = true)]
[return: MarshalAs(UnmanagedType.Bool)]
public static extern bool AdjustTokenPrivileges(IntPtr TokenHandle, [MarshalAs(UnmanagedType.Bool)]bool DisableAllPrivileges, ref TOKEN_PRIVILEGES NewState, uint BufferLength, IntPtr PreviousState, IntPtr ReturnLength);
static void Main(string[] args)
{
IntPtr currentProcessHandle = IntPtr.Zero;
IntPtr tokenHandle = IntPtr.Zero;
try
{
currentProcessHandle = System.Diagnostics.Process.GetCurrentProcess().Handle;
if (!OpenProcessToken(currentProcessHandle, TOKEN_ADJUST_PRIVILEGES | TOKEN_QUERY, out tokenHandle))
{
Console.WriteLine("OpenProcessToken failed: " + Marshal.GetLastWin32Error());
return;
}
// Get the required buffer size for token privileges
int tokenPrivilegesLength = 0;
TOKEN_PRIVILEGES tokenPrivileges = new TOKEN_PRIVILEGES();
AdjustTokenPrivileges(tokenHandle, false, ref tokenPrivileges, 0, IntPtr.Zero, IntPtr.Zero);
tokenPrivilegesLength = Marshal.SizeOf(tokenPrivileges);
// Allocate memory for token privileges
IntPtr tokenPrivilegesPtr = Marshal.AllocHGlobal(tokenPrivilegesLength);
Marshal.StructureToPtr(tokenPrivileges, tokenPrivilegesPtr, false);
// Enable all privileges
tokenPrivileges.PrivilegeCount = 1;
tokenPrivileges.Privileges = new LUID_AND_ATTRIBUTES[1];
LUID luid = new LUID();
if (!LookupPrivilegeValue(null, "SeDebugPrivilege", out luid))
{
Console.WriteLine("LookupPrivilegeValue failed: " + Marshal.GetLastWin32Error());
return;
}
tokenPrivileges.Privileges[0].Luid = luid;
tokenPrivileges.Privileges[0].Attributes = SE_PRIVILEGE_ENABLED;
Marshal.StructureToPtr(tokenPrivileges, tokenPrivilegesPtr, false);
if (!AdjustTokenPrivileges(tokenHandle, false, ref tokenPrivileges, tokenPrivilegesLength, IntPtr.Zero, IntPtr.Zero))
{
Console.WriteLine("AdjustTokenPrivileges failed: " + Marshal.GetLastWin32Error());
return;
}
int nameLength = MAX_NAME_LENGTH;
System.Text.StringBuilder nameBuilder = new System.Text.StringBuilder(nameLength);
bool success = LookupPrivilegeName(null, ref luid, nameBuilder, ref nameLength);
if (!success)
{
Console.WriteLine("LookupPrivilegeName failed: " + Marshal.GetLastWin32Error());
return;
}
Console.WriteLine("Enabled privileges:");
Console.WriteLine(nameBuilder.ToString());
}
finally
{
if (tokenHandle != IntPtr.Zero)
{
Marshal.FreeHGlobal(tokenHandle);
}
if (currentProcessHandle != IntPtr.Zero)
{
Marshal.FreeHGlobal(currentProcessHandle);
}
}
}
}
```
这段代码会输出当前进程启用的权限列表。其中,LookupPrivilegeName 函数通过一个 LUID(本地唯一标识符)来获取权限名称。这个 LUID 可以通过 LookupPrivilegeValue 函数获取。这里的代码演示了如何启用 SeDebugPrivilege 来获取当前进程的所有权限。
相关推荐
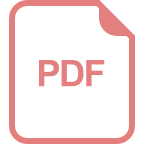
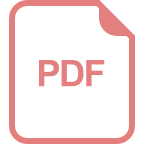














