能用c语言写一个贪吃蛇的代码吗
时间: 2023-06-20 11:08:12 浏览: 114
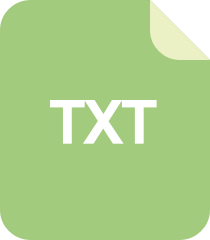
用c语言编写贪吃蛇程序

当然可以,以下是一个简单的贪吃蛇游戏的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#include <windows.h>
#define HEIGHT 20
#define WIDTH 40
int score = 0;
struct Snake {
int x;
int y;
struct Snake *next;
} *head, *tail;
struct Food {
int x;
int y;
} food;
void initMap(char map[HEIGHT][WIDTH]) {
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (i == 0 || i == HEIGHT - 1 || j == 0 || j == WIDTH - 1) {
map[i][j] = '#';
} else {
map[i][j] = ' ';
}
}
}
}
void printMap(char map[HEIGHT][WIDTH]) {
system("cls");
printf("Score: %d\n", score);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
printf("%c", map[i][j]);
}
printf("\n");
}
}
void createSnake() {
head = (struct Snake *) malloc(sizeof(struct Snake));
head->x = WIDTH / 2;
head->y = HEIGHT / 2;
head->next = NULL;
tail = head;
}
void createFood(char map[HEIGHT][WIDTH]) {
srand((unsigned) time(NULL));
while (1) {
int x = rand() % (WIDTH - 2) + 1;
int y = rand() % (HEIGHT - 2) + 1;
if (map[y][x] == ' ') {
food.x = x;
food.y = y;
map[food.y][food.x] = '@';
break;
}
}
}
void moveSnake(char map[HEIGHT][WIDTH], int dx, int dy) {
int newX = head->x + dx;
int newY = head->y + dy;
if (newX == food.x && newY == food.y) { // eat food
score++;
struct Snake *newHead = (struct Snake *) malloc(sizeof(struct Snake));
newHead->x = newX;
newHead->y = newY;
newHead->next = head;
head = newHead;
map[newY][newX] = '*';
createFood(map);
} else { // move forward
struct Snake *newHead = (struct Snake *) malloc(sizeof(struct Snake));
newHead->x = newX;
newHead->y = newY;
newHead->next = head;
head = newHead;
map[newY][newX] = '*';
map[tail->y][tail->x] = ' ';
struct Snake *tmp = tail;
tail = tail->next;
free(tmp);
}
}
int main() {
char map[HEIGHT][WIDTH];
initMap(map);
createSnake();
createFood(map);
printMap(map);
while (1) {
if (_kbhit()) {
char c = _getch();
if (c == 'w' || c == 'W') {
moveSnake(map, 0, -1);
} else if (c == 'a' || c == 'A') {
moveSnake(map, -1, 0);
} else if (c == 's' || c == 'S') {
moveSnake(map, 0, 1);
} else if (c == 'd' || c == 'D') {
moveSnake(map, 1, 0);
} else if (c == 'q' || c == 'Q') {
break;
}
} else {
moveSnake(map, 0, 0);
}
printMap(map);
Sleep(100);
}
return 0;
}
```
这个示例代码使用了简单的字符界面,使用wasd控制贪吃蛇的移动方向,每次吃到食物会增加分数并且贪吃蛇会变长,如果撞到墙或者自己的身体,游戏结束。
阅读全文
相关推荐





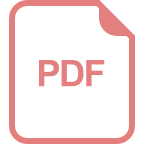
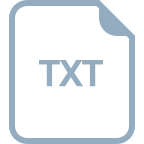
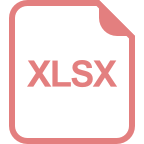
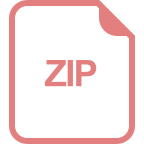
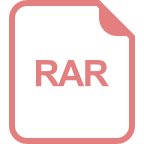
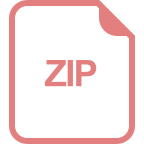
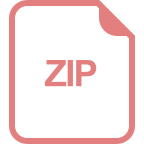
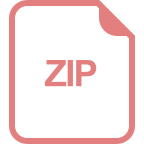
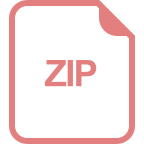