一个由tk按钮指令控制的封装成函数的pygame俄罗斯方块小游戏
时间: 2023-08-31 10:30:01 浏览: 67
### 回答1:
这里是一个简单的pygame俄罗斯方块小游戏,你可以使用tkinter按钮控制方块的移动和旋转。
首先,我们需要导入pygame和tkinter库:
```python
import pygame
import tkinter as tk
```
然后,我们定义一些常量和变量:
```python
# 游戏区域大小
WIDTH, HEIGHT = 300, 600
# 方块大小
BLOCK_SIZE = 30
# 游戏区域左上角坐标
TOP_LEFT_X, TOP_LEFT_Y = 50, 50
# 方块颜色
COLORS = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (255, 255, 255)]
# 方块形状
SHAPES = [[[1, 1, 1],
[0, 1, 0]],
[[2, 2],
[2, 2]],
[[0, 3, 3],
[3, 3, 0]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6, 0],
[0, 6, 6]],
[[0, 7, 0],
[7, 7, 7]]]
# 当前方块位置和形状
current_shape = SHAPES[0]
current_x = 4
current_y = 0
# 游戏区域
board = [[0 for _ in range(10)] for _ in range(20)]
# 游戏状态
game_over = False
```
接下来,我们定义一些函数来绘制游戏区域和方块:
```python
def draw_block(x, y, color):
pygame.draw.rect(screen, color, (TOP_LEFT_X + x * BLOCK_SIZE, TOP_LEFT_Y + y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def draw_board():
for y in range(len(board)):
for x in range(len(board[y])):
draw_block(x, y, COLORS[board[y][x]])
def draw_shape():
for y in range(len(current_shape)):
for x in range(len(current_shape[y])):
if current_shape[y][x] != 0:
draw_block(current_x + x, current_y + y, COLORS[current_shape[y][x]])
```
我们还需要定义一些函数来控制方块的移动和旋转:
```python
def move_left():
global current_x
if not game_over:
if check_collision(current_shape, current_x - 1, current_y):
return
current_x -= 1
def move_right():
global current_x
if not game_over:
if check_collision(current_shape, current_x + 1, current_y):
return
current_x += 1
def move_down():
global current_y
if not game_over:
if check_collision(current_shape, current_x, current_y + 1):
place_shape()
return
current_y += 1
def rotate_shape():
global current_shape
if not game_over:
new_shape = []
for i in range(len(current_shape[0])):
new_row = []
for row in current_shape:
new_row.append(row[i])
new_row.reverse()
new_shape.append(new_row)
if not check_collision(new_shape, current_x, current_y):
current_shape = new_shape
```
最后,我们定义一个主循环来处理游戏事件和更新游戏状态:
```python
def main_loop():
global game_over
clock = pygame.time.Clock()
while not game_over:
clock.tick(10)
screen.fill(COLORS[0])
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
move_left()
elif event.key == pygame.K_RIGHT:
move_right()
elif event.key == pygame.K_DOWN:
move_down()
elif event.key == pygame.K_UP:
rotate_shape()
# 绘制游戏区域和方块
draw_board()
draw_shape()
# 更新屏幕
pygame.display.update()
pygame.quit()
```
现在,我们可以把这些函数封装成一个函数,接受一个tkinter按钮作为参数来控制方块的移动和旋转:
```python
def tetris(button):
global game_over, current_shape, current_x, current_y, board
# 初始化pygame
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Tetris")
# 主循环
main_loop()
# 关闭pygame
pygame.quit()
def move_left():
global current_x
if not game_over:
if check_collision(current_shape, current_x - 1, current_y):
return
current_x -= 1
button.config(state=tk.DISABLED)
def move_right():
global current_x
if not game_over:
if check_collision(current_shape, current_x + 1, current_y):
return
current_x += 1
button.config(state=tk.DISABLED)
def move_down():
global current_y
if not game_over:
if check_collision(current_shape, current_x, current_y + 1):
place_shape()
return
current_y += 1
button.config(state=tk.DISABLED)
def rotate_shape():
global current_shape
if not game_over:
new_shape = []
for i in range(len(current_shape[0])):
new_row = []
for row in current_shape:
new_row.append(row[i])
new_row.reverse()
new_shape.append(new_row)
if not check_collision(new_shape, current_x, current_y):
current_shape = new_shape
button.config(state=tk.DISABLED)
def place_shape():
global current_shape, current_x, current_y, board, game_over
for y in range(len(current_shape)):
for x in range(len(current_shape[y])):
if current_shape[y][x] != 0:
board[current_y + y][current_x + x] = current_shape[y][x]
if current_y + y == 0:
game_over = True
clear_lines()
new_shape()
button.config(state=tk.NORMAL)
def clear_lines():
global board
new_board = []
for row in board:
if 0 not in row:
new_board.append([0 for _ in range(len(row))])
else:
new_board.append(row)
board = new_board
def new_shape():
global current_shape, current_x, current_y
current_shape = SHAPES[random.randint(0, len(SHAPES) - 1)]
current_x = 4
current_y = 0
if check_collision(current_shape, current_x, current_y):
game_over = True
def check_collision(shape, x, y):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0 and (y + i >= len(board) or x + j < 0 or x + j >= len(board[y])) or board[y + i][x + j] != 0:
return True
return False
def draw_block(x, y, color):
pygame.draw.rect(screen, color, (TOP_LEFT_X + x * BLOCK_SIZE, TOP_LEFT_Y + y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def draw_board():
for y in range(len(board)):
for x in range(len(board[y])):
draw_block(x, y, COLORS[board[y][x]])
def draw_shape():
for y in range(len(current_shape)):
for x in range(len(current_shape[y])):
if current_shape[y][x] != 0:
draw_block(current_x + x, current_y + y, COLORS[current_shape[y][x]])
def main_loop():
global game_over
clock = pygame.time.Clock()
while not game_over:
clock.tick(10)
screen.fill(COLORS[0])
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
move_left()
elif event.key == pygame.K_RIGHT:
move_right()
elif event.key == pygame.K_DOWN:
move_down()
elif event.key == pygame.K_UP:
rotate_shape()
# 绘制游戏区域和方块
draw_board()
draw_shape()
# 更新屏幕
pygame.display.update()
pygame.quit()
# 初始化游戏状态
game_over = False
current_shape = SHAPES[random.randint(0, len(SHAPES) - 1)]
current_x = 4
current_y = 0
board = [[0 for _ in range(10)] for _ in range(20)]
# 启动游戏循环
main_loop()
```
你可以使用以下代码来创建一个tkinter按钮来控制方块的移动和旋转:
```python
root = tk.Tk()
button = tk.Button(root, text='Move Left', command=lambda: move_left())
button.pack()
root.mainloop()
```
使用类似的方式,你可以创建其他按钮来控制方块的移动和旋转。
### 回答2:
这个由tk按钮指令控制的封装成函数的pygame俄罗斯方块小游戏非常具有趣味性和挑战性。游戏界面采用pygame库来绘制,通过tkinter提供的按钮指令来控制方块的下落和旋转。
游戏开始时,方块从顶部逐渐下落,玩家需要通过点击按钮来移动方块的位置以及旋转方块的形状。当方块堆积到底部或者无法再下落时,游戏结束,玩家可以选择重新开始或退出。
在游戏过程中,玩家需要根据方块的形状和当前的堆积情况来合理地安排方块的位置,以便消除填满的一行或多行方块。每次消除行动作,玩家将获得一定的得分,得分越高,游戏难度也随之增加。
游戏设计中,通过封装成函数的方式,使得游戏逻辑更加清晰可读,方便后续的维护和修改。同时,采用pygame库来绘制游戏界面,使得游戏具有更好的图形效果和交互体验。
总的来说,这个由tk按钮指令控制的封装成函数的pygame俄罗斯方块小游戏非常有吸引力。它结合了图形界面和游戏逻辑的设计,挑战玩家的思考和操作能力。无论是休闲娱乐还是培养反应能力都非常适合。
### 回答3:
俄罗斯方块是一款经典的游戏,通过使用tk按钮指令来控制游戏的几个方面,可以使游戏更加具有交互性,增加玩家的乐趣。这里我将介绍一个由tk按钮指令控制的封装成函数的pygame俄罗斯方块小游戏。
首先,我们需要导入相应的模块,包括pygame和tkinter。通过pygame创建游戏窗口,并初始化游戏的设置。然后,我们可以定义一些游戏所需的变量,比如方块的大小、形状和颜色等。
接下来,我们可以定义一些函数来处理游戏中的不同操作。例如,我们可以定义一个函数来生成新的俄罗斯方块,另一个函数来移动方块,还可以定义一个函数来判断方块是否满足消除条件等。这些函数可以被tk按钮指令调用,实现玩家对游戏的控制。
在游戏的主循环中,我们可以通过监听键盘事件来控制游戏的进行。同时,我们可以通过tk按钮指令来调用相应的函数,实现玩家对游戏的操作。例如,点击“左移”按钮时,我们可以调用移动方块函数,使方块向左移动一格。
最后,如果玩家完成了一行方块的堆叠,我们需要判断是否需要消除这一行,并调整上方方块的位置。这可以通过判断每一行方块的状态来实现。如果有一行方块都是满的,我们可以将这一行消除,并将上方方块往下移动一行。
综上所述,一个由tk按钮指令控制的封装成函数的pygame俄罗斯方块小游戏可以通过使用pygame和tkinter模块来实现。通过定义相应的函数和监听相应的事件,玩家可以通过按钮来控制游戏的进行。这样,游戏就更加具有互动性和趣味性。
相关推荐
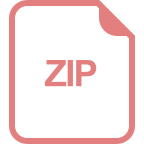
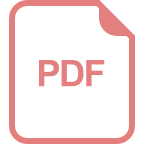


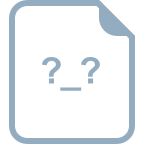
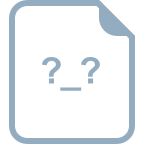
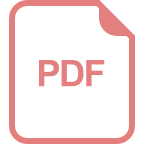
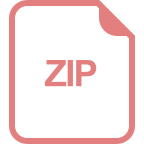
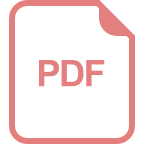
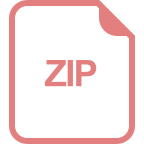
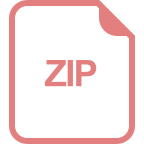
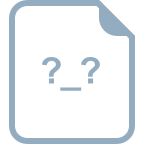
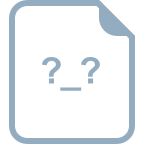
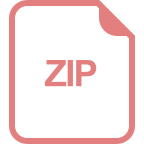
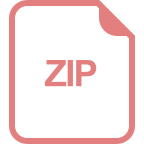
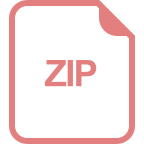