springboot树形结构案例
时间: 2023-10-13 13:26:38 浏览: 96
以下是一个简单的springboot树形结构案例:
首先,我们需要创建一个实体类,表示节点:
```java
public class Node {
private int id;
private String name;
private int parentId;
private List<Node> children = new ArrayList<>();
// 省略 getter 和 setter 方法
}
```
然后,我们需要创建一个服务类,用于获取树形结构数据:
```java
@Service
public class NodeService {
private List<Node> nodeList = new ArrayList<>();
@PostConstruct
public void init() {
// 初始化节点数据
nodeList.add(new Node(1, "节点1", 0));
nodeList.add(new Node(2, "节点2", 0));
nodeList.add(new Node(3, "节点3", 1));
nodeList.add(new Node(4, "节点4", 1));
nodeList.add(new Node(5, "节点5", 2));
nodeList.add(new Node(6, "节点6", 2));
nodeList.add(new Node(7, "节点7", 3));
nodeList.add(new Node(8, "节点8", 4));
nodeList.add(new Node(9, "节点9", 5));
}
public List<Node> getTree() {
List<Node> result = new ArrayList<>();
Map<Integer, Node> nodeMap = new HashMap<>();
for (Node node : nodeList) {
nodeMap.put(node.getId(), node);
}
for (Node node : nodeList) {
if (node.getParentId() == 0) {
result.add(node);
} else {
Node parent = nodeMap.get(node.getParentId());
parent.getChildren().add(node);
}
}
return result;
}
}
```
在上面的服务类中,我们使用一个Map来存储所有节点对象,然后遍历节点列表,将每个节点添加到其父节点的子节点列表中。如果节点的parentId为0,则该节点是根节点,直接添加到结果列表中。
最后,我们需要创建一个控制器类,用于接收请求并返回树形结构数据:
```java
@RestController
public class NodeController {
@Autowired
private NodeService nodeService;
@GetMapping("/tree")
public List<Node> getTree() {
return nodeService.getTree();
}
}
```
在上面的控制器类中,我们创建了一个GET请求,返回树形结构数据。
现在,我们可以运行该应用程序,并访问“/tree”路径来获取树形结构数据。
阅读全文
相关推荐
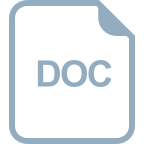
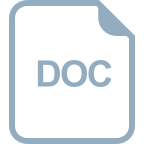
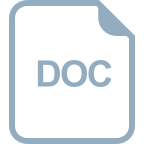
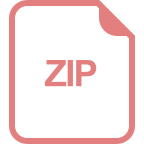
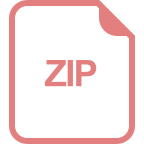
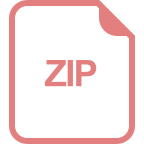
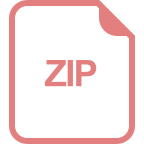
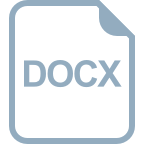
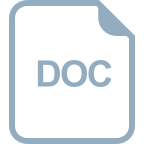
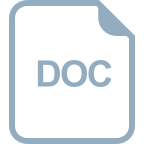
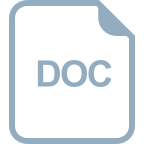
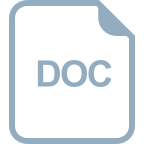
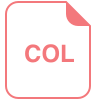
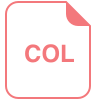
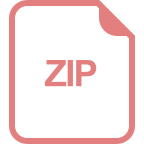
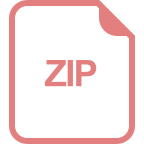