包含指针字段的结构体,生成一段C语言实现
时间: 2024-09-22 14:09:45 浏览: 58
在C语言中,你可以通过定义一个包含指针字段的结构体来创建一个动态数据结构。这通常用于存储指向其他内存位置的数据或引用。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个结构体,包含一个整型指针和一个整数值
typedef struct {
int* pointer_field; // 指向整数的指针
int value_field; // 结构体自身的值
} PointerStruct;
// 动态分配结构体实例并初始化指针
PointerStruct* create_struct(int* initial_value) {
PointerStruct* new_struct = (PointerStruct*)malloc(sizeof(PointerStruct));
if (new_struct) {
new_struct->pointer_field = initial_value;
new_struct->value_field = 0; // 初始化内部值
printf("Created a new struct with pointer pointing to %d\n", *initial_value);
} else {
printf("Memory allocation failed.\n");
}
return new_struct;
}
// 示例中释放结构体及其内容
void free_struct(PointerStruct* ptr) {
if (ptr && ptr->pointer_field) {
free(ptr->pointer_field); // 释放指针所指向的内容
free(ptr); // 释放结构体本身
}
}
int main() {
int value = 42;
PointerStruct* my_ptr = create_struct(&value);
// 使用结构体的指针访问值
printf("Value pointed by the pointer: %d\n", *my_ptr->pointer_field);
// 在这里可以修改指针所指向的内容
*my_ptr->pointer_field = 99;
printf("New value pointed by the pointer: %d\n", *my_ptr->pointer_field);
free_struct(my_ptr);
return 0;
}
```
在这个示例中,`create_struct`函数创建了一个新的`PointerStruct`实例,并将其`pointer_field`设置为传递的指针。`main`函数展示了如何使用这个结构体以及在其生命周期结束时如何释放内存。
阅读全文
相关推荐
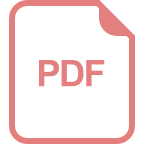
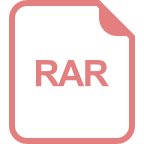
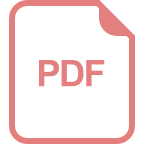
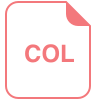
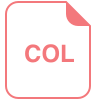


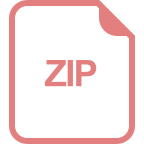
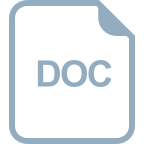
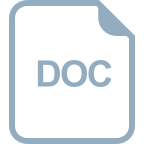
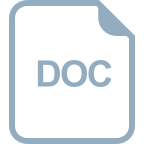
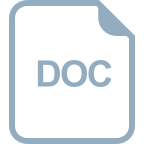
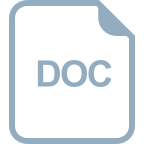
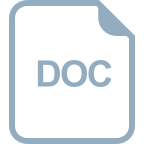
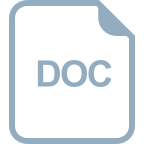



