使用C语言编写程序。使用指针操作结构体。实现学生和教师信息的输入、输出、修改等功能。
时间: 2024-10-19 10:09:44 浏览: 29
在C语言中,使用结构体(struct)可以方便地组织相关的数据,例如学生和教师的信息。首先,你需要定义一个结构体,如`Student`或`Teacher`,包含姓名、学号等属性:
```c
// 定义结构体
typedef struct {
char name[50];
int id;
// 添加其他必要的字段,比如年龄、专业等
} Student;
typedef struct {
char name[50];
int id; // 教师ID
char department[50]; // 所属部门
} Teacher;
```
接下来,你可以创建指向结构体的指针变量来存储数据,并通过指针进行操作。以下是基本的操作流程:
1. **输入**:
- 为用户分配内存空间,然后读取输入并初始化结构体实例。
```c
void input(Student* student) {
scanf("%s %d", student->name, &student->id);
// ... 其他输入字段
}
void input(Teacher* teacher) {
scanf("%s %d %s", teacher->name, &teacher->id, teacher->department);
// ... 其他输入字段
}
```
2. **输出**:
- 使用`printf`或其他输出函数显示结构体内容。
```c
void output(Student* student) {
printf("Name: %s, ID: %d\n", student->name, student->id);
}
void output(Teacher* teacher) {
printf("Name: %s, ID: %d, Department: %s\n", teacher->name, teacher->id, teacher->department);
}
```
3. **修改**:
- 调整结构体指针所指向的内容。
```c
void modify(Student* student, const char* new_name, int new_id) {
strcpy(student->name, new_name);
student->id = new_id;
// ... 更新其他字段
}
void modify(Teacher* teacher, const char* new_department) {
strcpy(teacher->department, new_department);
// ... 更新其他字段
}
```
完成以上功能后,你可以根据需要在主程序中调用这些函数。
阅读全文
相关推荐


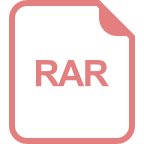









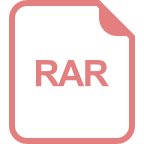
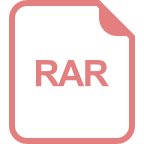



