java http请求获取tocken
时间: 2024-05-07 15:17:32 浏览: 123
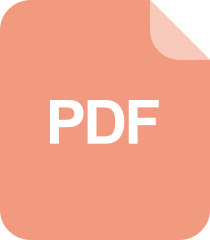
java http token请求代码实例

可以使用如下代码获取Java中的token:
```
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class TokenUtil {
public static String getToken(String clientId, String clientSecret) {
String plainCreds = clientId + ":" + clientSecret;
byte[] plainCredsBytes = plainCreds.getBytes(StandardCharsets.UTF_8);
String base64Creds = Base64.getEncoder().encodeToString(plainCredsBytes);
String tokenUrl = "http://your.auth.server/oauth/token"; //your auth server url
String grantType = "client_credentials"; //the type of grant
String scope = "read write"; //the scope of the access token
String url = tokenUrl + "?grant_type=" + grantType + "&scope=" + scope;
URL obj;
InputStream in = null;
try {
obj = new URL(url);
URLConnection conn = obj.openConnection();
HttpURLConnection httpConn = (HttpURLConnection) conn;
httpConn.setRequestMethod("POST");
httpConn.setRequestProperty("Authorization", "Basic " + base64Creds);
in = new BufferedInputStream(httpConn.getInputStream());
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
StringBuffer buffer = new StringBuffer();
String line;
while ((line = reader.readLine()) != null) {
buffer.append(line);
}
return buffer.toString();
} catch (Exception e) {
e.printStackTrace();
} finally {
if (in != null) {
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return null;
}
}
```
该方法使用了Basic认证方式,将client id和client secret进行base64编码后传递给token server。同时,也传递了`grant_type`和`scope`参数,用于指定授权方式和访问权限范围。在token server验证完成后,会返回一个JSON格式字符串,里面包括了access_token等信息。
阅读全文
相关推荐
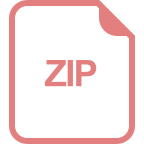
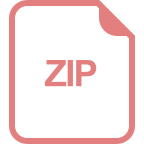
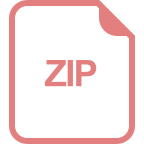






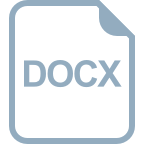
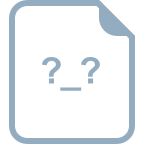
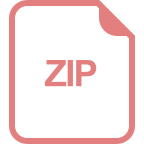
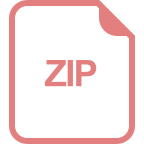
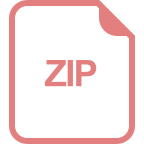