通过文件夹名获取所有文件索引
时间: 2023-07-04 08:30:26 浏览: 45
在 Linux 内核中,可以使用 `getdents` 系统调用来获取指定文件夹中的所有文件索引。该系统调用的原型如下:
```c
#include <dirent.h>
#include <unistd.h>
ssize_t getdents(unsigned int fd, struct linux_dirent *dirp, unsigned int count);
```
其中,`fd` 是指定文件夹的文件描述符;`dirp` 是一个指向 `linux_dirent` 结构体的指针,该结构体定义如下:
```c
struct linux_dirent {
unsigned long d_ino; /* Inode number */
unsigned long d_off; /* Offset to next linux_dirent */
unsigned short d_reclen; /* Length of this linux_dirent */
char d_name[]; /* Filename (null-terminated) */
/* length is actually (d_reclen - 2 -
offsetof(struct linux_dirent, d_name)) */
};
```
该结构体描述了一个文件夹中的一个文件信息,包括文件名、inode 号等信息。
`count` 参数指定了 `dirp` 缓冲区的大小,返回值为实际读取到的字节数,如果返回值为 0,则表示文件夹中没有文件。可以通过循环调用 `getdents` 函数来读取所有文件索引。
下面是一个简单的示例代码,用于获取指定文件夹中所有文件的 inode 号:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <unistd.h>
#include <fcntl.h>
struct linux_dirent {
unsigned long d_ino; /* Inode number */
unsigned long d_off; /* Offset to next linux_dirent */
unsigned short d_reclen; /* Length of this linux_dirent */
char d_name[]; /* Filename (null-terminated) */
};
int main(int argc, char **argv) {
DIR *dir;
struct dirent *dent;
struct linux_dirent *ldent;
char buf[1024];
int fd, nread;
if (argc != 2) {
printf("Usage: %s <directory>\n", argv[0]);
exit(1);
}
dir = opendir(argv[1]);
if (dir == NULL) {
perror("opendir");
exit(1);
}
fd = dirfd(dir);
if (fd < 0) {
perror("dirfd");
exit(1);
}
for (;;) {
nread = syscall(SYS_getdents, fd, buf, sizeof(buf));
if (nread == -1) {
perror("getdents");
exit(1);
}
if (nread == 0) break;
for (int bpos = 0; bpos < nread;) {
ldent = (struct linux_dirent *) (buf + bpos);
printf("%lu\n", ldent->d_ino);
bpos += ldent->d_reclen;
}
}
closedir(dir);
return 0;
}
```
注意,上述代码使用了 `syscall` 函数来调用 `getdents` 系统调用,这是因为 `getdents` 并没有被包含在标准 C 库中,需要使用系统调用来调用。
相关推荐
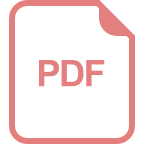
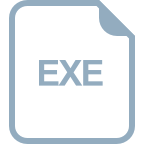














