opencv-python kmeans
时间: 2023-10-08 11:13:03 浏览: 101
K-means clustering is a popular unsupervised machine learning algorithm used for clustering data points into distinct groups or clusters. In OpenCV-Python, you can perform K-means clustering using the `kmeans` function from the `cv2` module.
Here is an example code snippet to demonstrate how to use K-means clustering in OpenCV-Python:
```python
import cv2
import numpy as np
# Load the image
image = cv2.imread('image.jpg')
# Reshape the image to a 2D array of pixels
pixels = image.reshape(-1, 3)
# Convert the pixel values to float32
pixels = np.float32(pixels)
# Define the criteria for the algorithm (number of iterations and epsilon)
criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 10, 1.0)
# Set the number of clusters
k = 5
# Perform K-means clustering
_, labels, centers = cv2.kmeans(pixels, k, None, criteria, 10, cv2.KMEANS_RANDOM_CENTERS)
# Convert the centers to uint8
centers = np.uint8(centers)
# Map each pixel to its corresponding cluster center
segmented_image = centers[labels.flatten()]
# Reshape the segmented image back to its original shape
segmented_image = segmented_image.reshape(image.shape)
# Display the original image and the segmented image
cv2.imshow('Original Image', image)
cv2.imshow('Segmented Image', segmented_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
Make sure to replace `'image.jpg'` with the path to your own image. The code reads an image, reshapes it into a 2D array of pixels, performs K-means clustering, maps each pixel to its corresponding cluster center, and displays the original image and the segmented image.
Feel free to ask if you have any further questions!
阅读全文
相关推荐
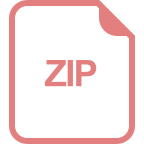
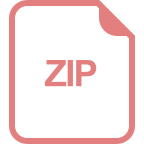
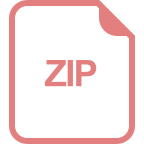
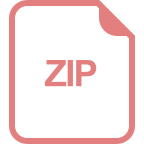
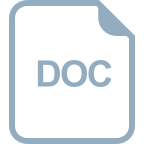
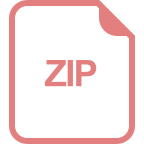
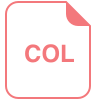
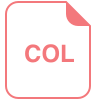


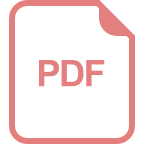
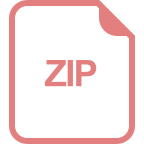
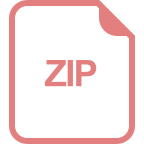
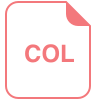


