Optimization Tips for OpenCV with Python: 10 Secrets to Enhance Image Processing Efficiency
发布时间: 2024-09-14 16:53:01 阅读量: 7 订阅数: 18 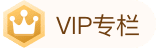
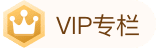
# Introduction to OpenCV and Python Image Processing
OpenCV (Open Source Computer Vision Library) is an open-source computer vision library that provides a wide range of image processing and computer vision algorithms. Its seamless integration with Python allows developers to easily build powerful image processing applications.
Python, as a high-level programming language, is renowned for its simplicity and readability. It offers a suite of tools and libraries for data processing, scientific computing, and machine learning. In combination with OpenCV, Python provides robust functionality and flexibility for image processing tasks.
This chapter will introduce the basics of OpenCV and Python image processing, including image data structures, basic image processing operations, and the integration between OpenCV and Python. By understanding these concepts, developers can lay the foundation for building efficient and scalable image processing applications.
# Theoretical Foundations of OpenCV and Python Optimization
### 2.1 Principles of Image Processing Algorithm Optimization
#### 2.1.1 Time and Space Complexity Analysis
When optimizing image processing algorithms, time complexity and space complexity are two key metrics. Time complexity measures the time required for the algorithm to execute, while space complexity measures the space required for the algorithm to execute.
- **Time complexity:** Usually denoted by the Big O notation, it represents the asymptotic growth rate of the algorithm's execution time. For example, O(n) indicates that the time complexity of the algorithm is directly proportional to the amount of input data n.
- **Space complexity:** Also denoted by the Big O notation, it represents the asymptotic growth rate of the space required for the algorithm's execution. For example, O(n) indicates that the space complexity of the algorithm is directly proportional to the amount of input data n.
Analyzing the time and space complexity of an algorithm can determine its efficiency and suitability.
#### 2.1.2 Parallelization and Multithreading Optimization
Parallelization and multithreading optimization are effective methods to improve the performance of image processing algorithms.
- **Parallelization:** Decompose the algorithm into multiple independent tasks and execute these tasks in parallel. This requires support from parallel hardware such as multicore CPUs or GPUs.
- **Multithreading:** Decompose the algorithm into multiple threads and execute these threads in parallel on a single CPU core. This requires a programming language and operating system that support multithreading operations.
Parallelization and multithreading optimization can significantly increase the execution speed of algorithms, especially when processing large datasets.
### 2.2 Python Code Optimization Tips
#### 2.2.1 Data Structures and Algorithm Selection
Choosing the appropriate Python data structures and algorithms is crucial for optimizing code performance.
- **Data structures:** Select suitable data structures for storing and operating on image data. For example, using NumPy arrays can improve the performance of array operations.
- **Algorithms:** Choose the algorithm that best fits the specific task. For example, using a quicksort algorithm can improve sorting performance.
#### 2.2.2 Code Conciseness and Readability
Writing concise and readable code not only aids maintenance but can also improve performance.
- **Conciseness:** Avoid unnecessary code and duplication.
- **Readability:** Use meaningful variable names, comments, and docstrings to enhance the comprehensibility of the code.
Concise and readable code is easier to understand and maintain, thereby reducing errors and improving performance.
# Practical Tips for OpenCV and Python Optimization
### 3.1 Image Preprocessing Optimization
#### 3.1.1 Image Scaling and Cropping
Image scaling and cropping are common operations in image preprocessing and can improve processing efficiency by reducing image size and removing unnecessary areas.
**Image Scaling**
```python
import cv2
# Load image
image = cv2.imread('image.jpg')
# Scale image to half size
scaled_image = cv2.resize(image, (image.shape[1] // 2, image.shape[0] // 2))
```
**Logical Analysis:**
* The `cv2.resize()` function is used for scaling images, where the first parameter is the input image, and the second parameter is the target size.
* `image.shape[1]` and `image.shape[0]` respectively obtain the width and height of the image, dividing by 2 indicates scaling to half size.
**Image Cropping**
```python
import cv2
# Load image
image = cv2.imread('image.jpg')
# Crop the central area of the image
cropped_image = image[100:300, 200:400]
```
**Logical Analysis:**
* `image[100:300, 200:400]` indicates cropping the area from row 100 to 300, and from column 200 to 400 of the image.
#### 3.1.2 Image Format Conversion
Image format conversion is also a common operation in image preprocessing. Different image formats have different storage methods and compression algorithms. Choosing the appropriate image format can opt
0
0
相关推荐
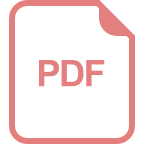
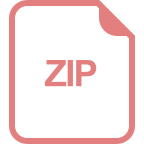
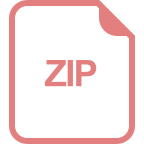





