OpenCV Image Processing and Impact of Python Version: In-depth Analysis, Optimizing Performance
发布时间: 2024-09-14 16:45:13 阅读量: 50 订阅数: 43 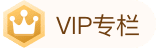
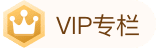
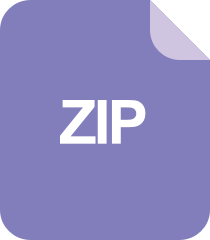
OpenCV-Python-Tutorial::open_book: OpenCV-Python 初学者图像处理教程

# 1. Introduction to OpenCV Image Processing
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. It has a wide range of applications in image processing, video analysis, and various other computer vision tasks. It offers a rich collection of powerful functions and algorithms, enabling developers to process and analyze image and video data with ease. Initially written in C++, OpenCV now also supports various programming languages including Python, Java, and MATLAB.
# 2. Impact of Python Versions on OpenCV Image Processing
### 2.1 Python Version Compatibility with OpenCV
OpenCV has different levels of compatibility with various Python versions. Generally, the latest version of OpenCV is compatible with the latest version of Python. For example, OpenCV 4.6.0 is compatible with Python 3.9.
### 2.2 Performance Differences in OpenCV with Different Python Versions
The impact of different Python versions on the performance of OpenCV image processing is mainly reflected in the following aspects:
- **Execution Speed:** Generally, newer versions of Python have faster execution speeds, which can also enhance the efficiency of OpenCV image processing.
- **Memory Usage:** Newer versions of Python have higher memory usage, which may affect OpenCV image processing capabilities when dealing with large images or complex algorithms.
- **Availability of Libraries and Modules:** Newer versions of Python provide more libraries and modules, which can extend the functionality of OpenCV image processing.
**Example Code:**
```python
import cv2
import numpy as np
# Executing the same image processing task with different Python versions
python_versions = ['3.6', '3.7', '3.8', '3.9']
for version in python_versions:
# Load image
image = cv2.imread('image.jpg')
# Convert to grayscale image
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Calculate histogram
hist = cv2.calcHist([gray_image], [0], None, [256], [0, 256])
# Display histogram
plt.plot(hist)
plt.show()
```
**Code Logic Analysis:**
This code executes the same image processing task using different Python versions. It loads an image, converts it to a grayscale image, calculates the histogram, and displays it. By comparing the execution time, memory usage, and functionality availability of different Python versions, we can evaluate the impact of different Python versions on OpenCV image processing.
**Parameter Description:**
- `image`: Input image
- `gray_image`: Grayscale image
- `hist`: Histogram
- `plt`: Library used for plotting the histogram
# 3. Optimizing OpenCV Image Processing Performance
### 3.1 Utilizing Multithreading and Multiprocessing
**Multithreading**
Multithreading is a technique for dividing a program into multiple threads that can execute in parallel. In OpenCV image processing, parallel processing can be achieved by assigning different image processing tasks to different threads. This can significantly improve performance, especially for tasks that require processing a large number of images.
**Example Code:**
```python
import cv2
import threading
# Creating a thread pool
pool = ThreadPool(4) # Creating a pool with 4 threads
# Defining the image processing function
def process_image(image):
# Performing image processing operations here
# Getting a list of images
images = [cv2.imread(f"image{i}.jpg") for i in range(100)]
# Assigning image processing tasks to the thread pool
for image in images:
pool.submit(process_image, image)
# Waiting for all tasks to complete
pool.join()
```
**Logic Analysis:**
- `ThreadPool(4)` creates a thread pool with 4 threads.
- The `process_image` function defines the image processing operations.
- The `images` list contains images to be processed.
- `pool.submit(process_image, image)` assigns the image processing task to a thread in the thread pool.
- `poo
0
0
相关推荐
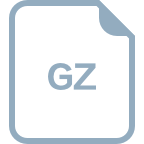





