The Impact of OpenCV and Python Versions in Computer Vision Applications: A Case Study Exploring Practical Value
发布时间: 2024-09-14 16:55:19 阅读量: 21 订阅数: 27 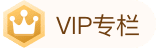
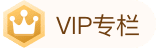
# 1. The Foundation of OpenCV and Python in Computer Vision
OpenCV (Open Source Computer Vision Library) and Python are widely used tools in the field of computer vision. OpenCV is a cross-platform open-source library that provides a wide range of image processing and computer vision algorithms. Python is a high-level programming language known for its ease of use and extensive libraries.
In computer vision, the combination of OpenCV and Python offers powerful capabilities. OpenCV provides low-level image processing and computer vision algorithms, while Python provides high-level programming features such as scripting, data analysis, and visualization. This combination enables developers to easily build and deploy computer vision applications.
# 2. Analysis of OpenCV and Python Version Differences
### 2.1 Language Feature Comparison
#### 2.1.1 Data Structures and Type Systems
OpenCV and Python have significant differences in data structures and type systems. OpenCV, developed using the C++ language, supports a variety of data structures, including arrays, matrices, images, and video sequences. Python is a dynamically typed language that supports data structures such as lists, tuples, dictionaries, and sets.
**Code Block:**
```python
import numpy as np
# In OpenCV, images are represented as multi-dimensional arrays
image = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# In Python, lists are represented as one-dimensional arrays
list_data = [1, 2, 3, 4, 5, 6]
```
**Logical Analysis:**
Images in OpenCV are represented as multi-dimensional arrays, while lists in Python are represented as one-dimensional arrays. OpenCV arrays provide direct access to image pixels, whereas Python lists are better suited for storing one-dimensional data.
#### 2.1.2 Control Flow and Functional Programming
OpenCV and Python also differ in control flow and functional programming. OpenCV uses C++ control flow structures, including if-else statements, loops, and switch statements. Python supports object-oriented programming and functional programming, offering features such as lambda expressions, generators, and list comprehensions.
**Code Block:**
```python
# Control flow in OpenCV
if image.shape[0] > 100:
print("Image height is greater than 100")
# Functional programming in Python
filtered_list = list(filter(lambda x: x > 3, list_data))
```
**Logical Analysis:**
OpenCV uses traditional control flow structures, whereas Python supports functional programming, allowing operations on data using lambda expressions and generators. Functional programming provides more concise and readable code.
### 2.2 Library and Toolset Comparison
#### 2.2.1 Image Processing and Analysis
Both OpenCV and Python offer extensive libraries for image processing and analysis. OpenCV contains functions for image reading, conversion, enhancement, segmentation, and object detection. Python provides libraries such as Pillow, Scikit-Image, and OpenCV-Python for image processing and analysis.
**Code Block:**
```python
import cv2
# Image conversion in OpenCV
image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Image scaling in Pillow
from PIL import Image
image = Image.open("image.jpg").resize((200, 200))
```
**Logical Analysis:**
OpenCV provides a wide range of image processing functions, whereas Python libraries offer higher-level image processing capabilities such as image scaling and conversion.
#### 2.2.2 Machine Learning and Deep Learning
Both OpenCV and Python support machine learning and deep learning. OpenCV includes modules for machine learning and deep learning, such as SVM, random forests, and neural networks. Python offers libraries such as TensorFlow, Keras, and PyTorch for deep learning and machine learning.
**Code Block:**
```python
import tensorflow as tf
# Neural network model in TensorFlow
model = tf.keras.models.Sequential([
tf.keras.layers.D
```
0
0
相关推荐
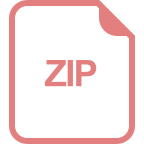
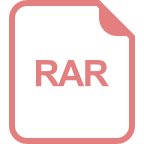
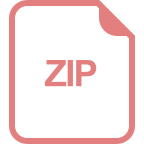
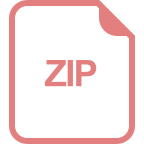
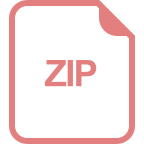
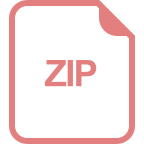
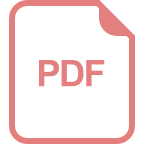
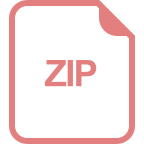
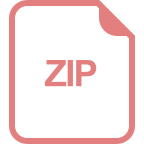