java 查询树结构部门关联人员
时间: 2023-10-27 21:53:29 浏览: 131
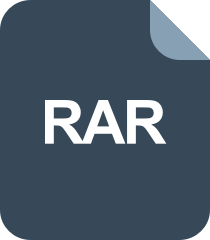
flex人力资源树例子,部门+人员结构图,使用tree+checkbox实现

对于 Java 查询树结构部门关联人员,您可以使用递归算法来实现。下面是一个简单的示例代码:
首先,您需要定义一个部门类 `Department`,其中包含部门的名称、所属上级部门以及部门下的员工列表等属性。然后,您可以使用一个树形结构来组织部门之间的关系。
```java
import java.util.ArrayList;
import java.util.List;
public class Department {
private String name;
private Department parent;
private List<Employee> employees;
public Department(String name, Department parent) {
this.name = name;
this.parent = parent;
this.employees = new ArrayList<>();
}
public String getName() {
return name;
}
public Department getParent() {
return parent;
}
public List<Employee> getEmployees() {
return employees;
}
// 添加员工
public void addEmployee(Employee employee) {
employees.add(employee);
}
}
```
然后,您可以定义一个员工类 `Employee`,其中包含员工的姓名等属性。
```java
public class Employee {
private String name;
public Employee(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
```
接下来,我们可以创建一棵部门树,并添加部门和员工信息。
```java
public class Main {
public static void main(String[] args) {
// 创建根部门
Department root = new Department("总部", null);
// 创建子部门
Department department1 = new Department("部门1", root);
Department department2 = new Department("部门2", root);
// 创建员工
Employee employee1 = new Employee("员工1");
Employee employee2 = new Employee("员工2");
Employee employee3 = new Employee("员工3");
Employee employee4 = new Employee("员工4");
// 将员工添加到部门中
department1.addEmployee(employee1);
department1.addEmployee(employee2);
department2.addEmployee(employee3);
department2.addEmployee(employee4);
// 查询树结构部门关联人员
List<Employee> allEmployees = getAllEmployees(root);
for (Employee employee : allEmployees) {
System.out.println(employee.getName());
}
}
// 递归查询树结构部门关联人员
public static List<Employee> getAllEmployees(Department department) {
List<Employee> allEmployees = new ArrayList<>();
// 添加当前部门的员工列表
allEmployees.addAll(department.getEmployees());
// 递归查询子部门的员工列表
List<Department> childDepartments = getChildDepartments(department);
for (Department childDepartment : childDepartments) {
allEmployees.addAll(getAllEmployees(childDepartment));
}
return allEmployees;
}
// 获取子部门列表
public static List<Department> getChildDepartments(Department department) {
List<Department> childDepartments = new ArrayList<>();
// TODO: 根据实际情况获取子部门列表
return childDepartments;
}
}
```
在上述示例中,我们创建了一个包含树形结构部门和员工信息的部门树。然后,通过调用 `getAllEmployees` 方法,可以递归地获取所有部门关联的人员信息。您可以根据实际情况修改 `getChildDepartments` 方法,获取子部门列表。
阅读全文
相关推荐
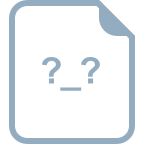
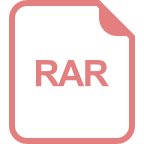
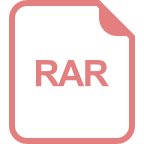
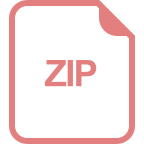
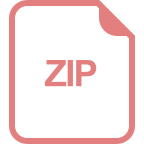
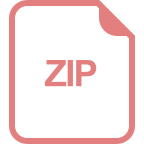
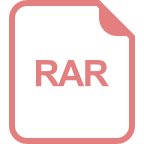
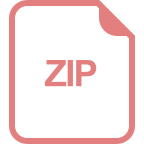
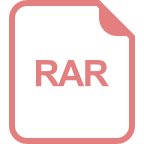
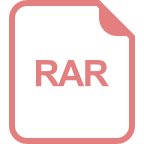
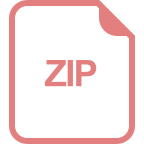
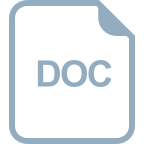
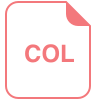
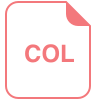
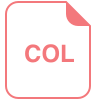
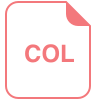
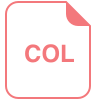
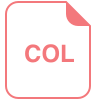