如何使用Pandas仅保留DataFrame中的特定两列数据?
时间: 2024-11-24 15:43:04 浏览: 50
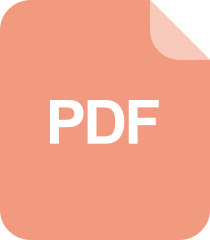
如何更改 pandas dataframe 中两列的位置
在Pandas中,你可以通过选择性地传递列名或列索引来保留DataFrame中的特定两列。如果你有列名,可以这样做:
```python
import pandas as pd
# 假设df是一个DataFrame
selected_columns = ['column1', 'column2'] # 替换为你的列名
new_df = df[selected_columns]
```
如果你已经有了列索引,可以直接使用它们:
```python
new_df = df.iloc[:, [index_of_col1, index_of_col2]]
```
在这里,`index_of_col1` 和 `index_of_col2` 是你想保留的列的相应索引。
如果你想动态指定列,可以使用条件筛选:
```python
column_names = df.columns.tolist() # 获取所有列名
specific_cols = ['column1', 'column2']
new_df = df[[name in specific_cols for name in column_names]]
```
这将返回一个新的DataFrame,只包含你在列表中指定的列。
阅读全文
相关推荐
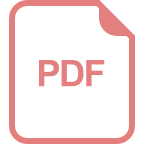
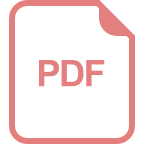






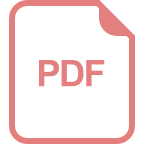
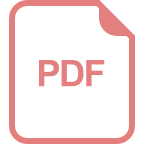
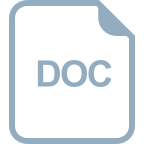
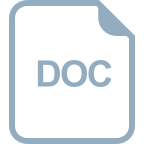
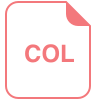
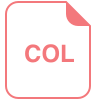


