基于tensorflow的完整最大均值差异图像分类代码
时间: 2023-06-16 20:02:30 浏览: 112
以下是使用 TensorFlow 完整最大均值差异(Max-Mean-Diff,MMD)图像分类的代码示例:
首先,导入必要的库:
``` python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
from tensorflow.keras.datasets import cifar10
```
然后,加载 CIFAR-10 数据集:
``` python
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
```
接着,对数据进行预处理:
``` python
x_train = x_train / 255.0
x_test = x_test / 255.0
y_train = tf.keras.utils.to_categorical(y_train, num_classes=10)
y_test = tf.keras.utils.to_categorical(y_test, num_classes=10)
```
定义 MMD 损失函数:
``` python
def maximum_mean_discrepancy(x, y, kernel=rbf_kernel):
cost = tf.reduce_mean(kernel(x, x))
cost += tf.reduce_mean(kernel(y, y))
cost -= 2 * tf.reduce_mean(kernel(x, y))
cost = tf.where(cost > 0, cost, 0, name='mmd_loss')
return cost
def rbf_kernel(x, y, gamma=0.05):
K_xx = tf.exp(-gamma * tf.square(tf.norm(x - x, ord='euclidean', axis=-1)))
K_yy = tf.exp(-gamma * tf.square(tf.norm(y - y, ord='euclidean', axis=-1)))
K_xy = tf.exp(-gamma * tf.square(tf.norm(x - y, ord='euclidean', axis=-1)))
return K_xx + K_yy - 2 * K_xy
```
定义模型:
``` python
def create_model():
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3,3), activation='relu', padding='same', input_shape=(32,32,3)),
tf.keras.layers.BatchNormalization(),
tf.keras.layers.Conv2D(32, (3,3), activation='relu', padding='same'),
tf.keras.layers.BatchNormalization(),
tf.keras.layers.MaxPooling2D((2,2)),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Conv2D(64, (3,3), activation='relu', padding='same'),
tf.keras.layers.BatchNormalization(),
tf.keras.layers.Conv2D(64, (3,3), activation='relu', padding='same'),
tf.keras.layers.BatchNormalization(),
tf.keras.layers.MaxPooling2D((2,2)),
tf.keras.layers.Dropout(0.3),
tf.keras.layers.Conv2D(128, (3,3), activation='relu', padding='same'),
tf.keras.layers.BatchNormalization(),
tf.keras.layers.Conv2D(128, (3,3), activation='relu', padding='same'),
tf.keras.layers.BatchNormalization(),
tf.keras.layers.MaxPooling2D((2,2)),
tf.keras.layers.Dropout(0.4),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
return model
```
编译模型并训练:
``` python
model = create_model()
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
model.compile(optimizer=optimizer, loss='categorical_crossentropy', metrics=['accuracy'])
history = model.fit(x_train, y_train, epochs=50, validation_data=(x_test, y_test))
```
评估模型:
``` python
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
最后,绘制训练和验证准确率和损失函数的变化图:
``` python
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label = 'val_accuracy')
plt.plot(history.history['loss'], label = 'loss')
plt.plot(history.history['val_loss'], label = 'val_loss')
plt.xlabel('Epoch')
plt.ylabel('Accuracy/Loss')
plt.ylim([0, 1])
plt.legend(loc='lower right')
plt.show()
```
阅读全文
相关推荐
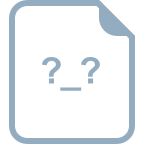



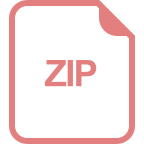
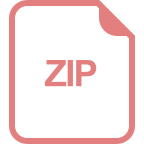
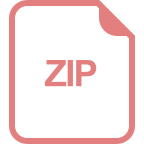
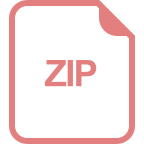
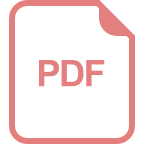
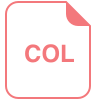
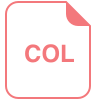
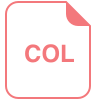
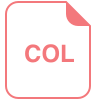
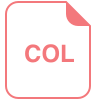
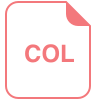
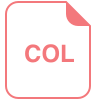
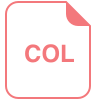