android studio怎么编写二级listview
时间: 2023-07-05 15:26:53 浏览: 72
在 Android Studio 中,可以使用 ExpandableListView 控件来实现二级列表。
以下是实现二级列表的步骤:
1. 在布局文件中添加 ExpandableListView 控件:
```xml
<ExpandableListView
android:id="@+id/expandableListView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
2. 创建一个 Adapter 类来提供数据和视图:
```java
public class MyExpandableListAdapter extends BaseExpandableListAdapter {
private Context context;
private List<String> groupList;
private Map<String, List<String>> childMap;
public MyExpandableListAdapter(Context context, List<String> groupList, Map<String, List<String>> childMap) {
this.context = context;
this.groupList = groupList;
this.childMap = childMap;
}
@Override
public int getGroupCount() {
return groupList.size();
}
@Override
public int getChildrenCount(int groupPosition) {
String groupName = groupList.get(groupPosition);
List<String> childList = childMap.get(groupName);
return childList.size();
}
@Override
public Object getGroup(int groupPosition) {
return groupList.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
String groupName = groupList.get(groupPosition);
List<String> childList = childMap.get(groupName);
return childList.get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
TextView textView = new TextView(context);
textView.setText(groupList.get(groupPosition));
textView.setPadding(100, 0, 0, 0);
textView.setTextSize(20);
return textView;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
TextView textView = new TextView(context);
String groupName = groupList.get(groupPosition);
List<String> childList = childMap.get(groupName);
textView.setText(childList.get(childPosition));
textView.setPadding(150, 0, 0, 0);
textView.setTextSize(16);
return textView;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
```
3. 在 Activity 中获取 ExpandableListView 控件的实例,并设置 Adapter:
```java
ExpandableListView expandableListView = findViewById(R.id.expandableListView);
List<String> groupList = new ArrayList<>();
groupList.add("Group 1");
groupList.add("Group 2");
Map<String, List<String>> childMap = new HashMap<>();
List<String> childList1 = new ArrayList<>();
childList1.add("Child 1-1");
childList1.add("Child 1-2");
childList1.add("Child 1-3");
childMap.put("Group 1", childList1);
List<String> childList2 = new ArrayList<>();
childList2.add("Child 2-1");
childList2.add("Child 2-2");
childMap.put("Group 2", childList2);
MyExpandableListAdapter adapter = new MyExpandableListAdapter(this, groupList, childMap);
expandableListView.setAdapter(adapter);
```
这样就可以在 Android 应用中实现二级列表了。如果需要更多的样式和交互效果,可以在 Adapter 中进行定制。
相关推荐
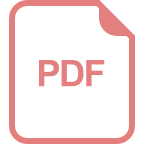
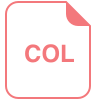













