用c语言实现大地坐标与空间坐标的相互转换
时间: 2023-09-14 12:07:38 浏览: 55
这里提供一种简单的实现方法:
1. 定义结构体表示大地坐标和空间坐标:
```c
typedef struct {
double longitude; // 经度,单位为度
double latitude; // 纬度,单位为度
double height; // 高度,单位为米
} GeodeticCoord;
typedef struct {
double x; // X轴坐标,单位为米
double y; // Y轴坐标,单位为米
double z; // Z轴坐标,单位为米
} SpaceCoord;
```
2. 实现大地坐标转空间坐标的函数,使用WGS84椭球模型计算:
```c
#define a 6378137.0 // WGS84椭球长半轴,单位为米
#define b 6356752.3142 // WGS84椭球短半轴,单位为米
#define e2 0.0066943799 // WGS84椭球第一偏心率的平方
void geodetic_to_space(const GeodeticCoord* geodetic, SpaceCoord* space) {
// 计算第一卯酉圈曲率半径
double N = a / sqrt(1.0 - e2 * sin(geodetic->latitude) * sin(geodetic->latitude));
// 计算空间直角坐标
space->x = (N + geodetic->height) * cos(geodetic->latitude) * cos(geodetic->longitude);
space->y = (N + geodetic->height) * cos(geodetic->latitude) * sin(geodetic->longitude);
space->z = (N * (1.0 - e2) + geodetic->height) * sin(geodetic->latitude);
}
```
3. 实现空间坐标转大地坐标的函数,使用迭代法求解:
```c
#define max_iter 10 // 迭代次数
#define eps 1e-10 // 精度要求
void space_to_geodetic(const SpaceCoord* space, GeodeticCoord* geodetic) {
double p = sqrt(space->x * space->x + space->y * space->y);
double theta = atan2(space->z * a, p * b);
double lat_old = atan2(space->z + e2 * b * pow(sin(theta), 3), p - e2 * a * pow(cos(theta), 3));
double lat_new = 0.0;
int i;
for (i = 0; i < max_iter; ++i) {
double N = a / sqrt(1.0 - e2 * sin(lat_old) * sin(lat_old));
lat_new = atan2(space->z + N * e2 * sin(lat_old), p);
if (fabs(lat_new - lat_old) < eps) {
break;
}
lat_old = lat_new;
}
geodetic->longitude = atan2(space->y, space->x);
geodetic->latitude = lat_new;
geodetic->height = p / cos(lat_new) - N;
}
```
这样就可以通过调用这两个函数实现大地坐标与空间坐标的相互转换了。需要注意的是,上述代码中使用的是WGS84椭球模型,实际应用中可能需要根据具体情况选择不同的椭球模型。
阅读全文
相关推荐
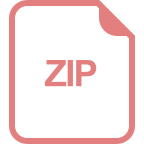
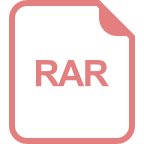
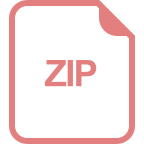
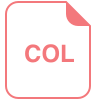
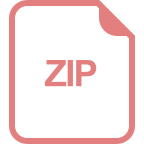
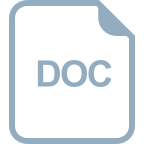
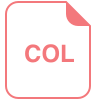
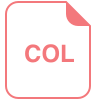




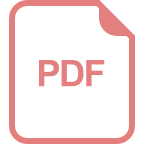
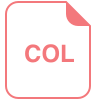
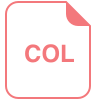
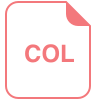


