二分查找份文件编写 c++
时间: 2023-07-23 14:00:08 浏览: 88
下面是一个使用二分查找算法进行搜索的C++代码示例,将二分查找的功能封装在一个单独的文件中:
`binary_search.h` 文件:
```cpp
#ifndef BINARY_SEARCH_H
#define BINARY_SEARCH_H
#include <vector>
template <typename T>
int binarySearch(const std::vector<T>& arr, T target) {
int left = 0;
int right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // 表示未找到目标元素
}
#endif // BINARY_SEARCH_H
```
`main.cpp` 文件:
```cpp
#include <iostream>
#include <vector>
#include "binary_search.h"
int main() {
std::vector<int> nums = {1, 3, 5, 7, 9, 11};
int target = 7;
int result = binarySearch(nums, target);
if (result != -1) {
std::cout << "目标元素 " << target << " 的索引位置为: " << result << std::endl;
} else {
std::cout << "未找到目标元素 " << target << std::endl;
}
return 0;
}
```
在这个示例中,我们将二分查找算法封装在 `binary_search.h` 文件中。这个文件定义了一个模板函数 `binarySearch`,接受一个类型为 `T` 的向量 `arr` 和要查找的目标元素 `target`。在 `main.cpp` 文件中,我们包含了 `binary_search.h` 头文件,并在 `main` 函数中调用 `binarySearch` 函数来进行查找。注意,为了使用 `binarySearch` 函数,需要将 `binary_search.h` 和 `main.cpp` 放在同一个目录下,并进行编译链接。
这样的组织方式使得代码更加模块化和可维护,可以方便地在其他项目中重用二分查找的功能。
阅读全文
相关推荐
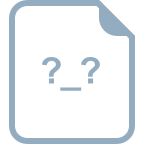
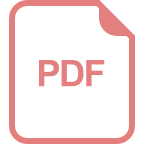
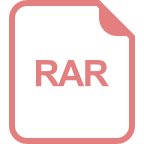
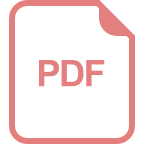
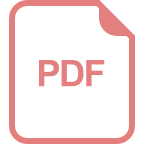
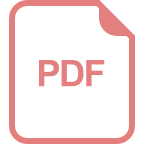
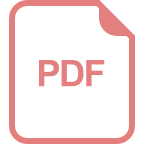
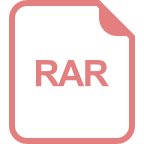
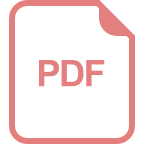
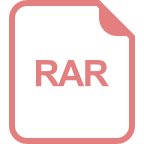
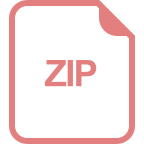
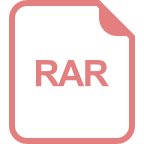
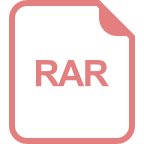
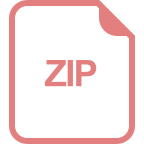
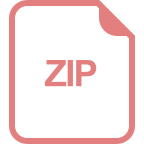
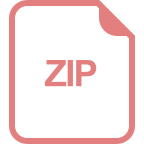
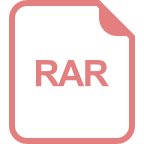