不使用LINQ查询和操作集合 改进代码 namespace SandwichCalories { class Program { static void Main(string[] args) { // sandwich ingredients and their associated calories Dictionary<string, int> ingredients = new Dictionary<string, int>() { { "Bread", 100 }, { "Ham", 150 }, { "Lettuce", 10 }, { "Tomato", 20 }, { "Mayonnaise", 50 }, { "Cheese", 120 } }; // prompt user for calorie range Console.Write("Enter minimum calories: "); int min_calories = int.Parse(Console.ReadLine()); Console.Write("Enter maximum calories: "); int max_calories = int.Parse(Console.ReadLine()); // calculate the minimum and maximum calories for the sandwich int min_sandwich_calories = 2 * ingredients["Bread"] + ingredients.Values.Min() * 2; int max_sandwich_calories = 2 * ingredients["Bread"] + ingredients.Values.Max() * 2; // check if the calorie range is valid if (max_calories < min_sandwich_calories) { Console.WriteLine("Sorry, it is impossible to create a sandwich within the given calorie range."); } else { // create the sandwich List<string> sandwich = new List<string> { "Bread", "Bread" }; int sandwich_calories = 2 * ingredients["Bread"]; while (sandwich_calories < min_calories) { // add random ingredient string ingredient = ingredients.Keys.ElementAt(new Random().Next(ingredients.Count)); sandwich.Add(ingredient); sandwich_calories += ingredients[ingredient]; } while (sandwich_calories <= max_calories) { // add random ingredient string ingredient = ingredients.Keys.ElementAt(new Random().Next(ingredients.Count)); // check if the ingredient is the same as the previous one if (sandwich.Count >= 3 && ingredient == sandwich[sandwich.Count - 2]) { continue; } sandwich.Add(ingredient); sandwich_calories += ingredients[ingredient]; // check if the sandwich is already at the maximum calorie limit if (sandwich_calories == max_sandwich_calories) { break; } } // add the last slice of bread sandwich.Add("Bread"); // print the sandwich and its total calories Console.WriteLine("Your sandwich: " + string.Join(", ", sandwich)); Console.WriteLine("Total calories: " + sandwich_calories); } } } }
时间: 2024-03-22 09:39:47 浏览: 45
One possible way to improve the code without using LINQ and manipulating collections is to simplify the while loop that adds random ingredients to the sandwich. Instead of generating a random index for the keys in the dictionary and then checking if the ingredient has already been added, we can use a random order of the keys and simply iterate over them until we reach the desired calorie limit:
```
// create the sandwich
List<string> sandwich = new List<string> { "Bread", "Bread" };
int sandwich_calories = 2 * ingredients["Bread"];
// add random ingredients until we reach the minimum calorie limit
List<string> random_ingredients = new List<string>(ingredients.Keys);
random_ingredients.Remove("Bread"); // exclude bread from random selection
random_ingredients.Shuffle(); // shuffle the order of the keys
foreach (string ingredient in random_ingredients) {
sandwich.Add(ingredient);
sandwich_calories += ingredients[ingredient];
if (sandwich_calories >= min_calories) {
break;
}
}
// add more random ingredients until we reach the maximum calorie limit
random_ingredients.Shuffle(); // shuffle the order again
foreach (string ingredient in random_ingredients) {
// check if the ingredient is the same as the previous one
if (sandwich.Count >= 3 && ingredient == sandwich[sandwich.Count - 2]) {
continue;
}
sandwich.Add(ingredient);
sandwich_calories += ingredients[ingredient];
// check if the sandwich is already at the maximum calorie limit
if (sandwich_calories >= max_calories || sandwich_calories == max_sandwich_calories) {
break;
}
}
```
The `Shuffle` method is a custom extension method that randomly shuffles the order of the elements in a list, which can be defined as follows:
```
public static class ListExtensions {
private static readonly Random random = new Random();
public static void Shuffle<T>(this IList<T> list) {
for (int i = list.Count - 1; i > 0; i--) {
int j = random.Next(i + 1);
T temp = list[j];
list[j] = list[i];
list[i] = temp;
}
}
}
```
By using a shuffled list of keys instead of generating a random index for each iteration, we can ensure that each ingredient is added to the sandwich at most once, without the need to check for duplicates. This also eliminates the possibility of an infinite loop if the same ingredient is randomly selected multiple times.
阅读全文
相关推荐
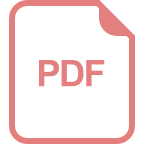
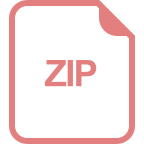
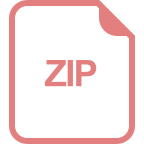
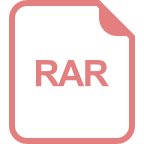
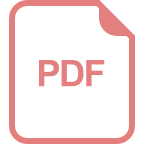
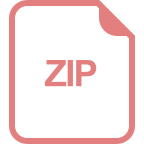
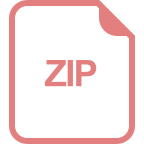
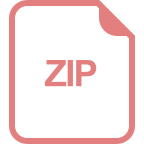
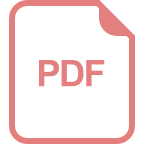
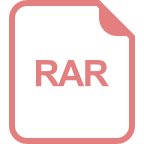
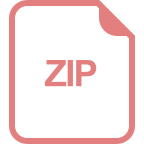
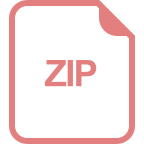
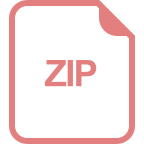
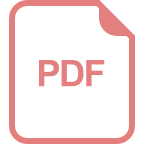
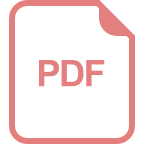