httpclient 文件上传工具类
时间: 2023-06-20 12:08:53 浏览: 125
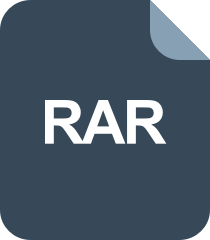
java httpclient https或http及文件中转上传工具类
以下是一个示例的 HttpClient 文件上传工具类,可以帮助你上传文件:
```java
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.FileUtils;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class HttpClientFileUploader {
public static void uploadFile(String url, File file) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody("file", file, ContentType.MULTIPART_FORM_DATA, file.getName());
HttpEntity multipart = builder.build();
httpPost.setEntity(multipart);
CloseableHttpResponse response = httpClient.execute(httpPost);
try {
HttpEntity responseEntity = response.getEntity();
if (responseEntity != null) {
String responseString = EntityUtils.toString(responseEntity, StandardCharsets.UTF_8);
System.out.println(responseString);
}
} finally {
response.close();
}
}
public static void main(String[] args) throws IOException {
File file = new File("path/to/file.txt");
String url = "http://example.com/upload";
uploadFile(url, file);
}
}
```
此示例使用 Apache HttpClient 库。请确保将其添加到项目依赖项中。
阅读全文
相关推荐
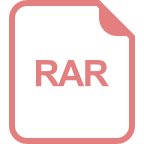
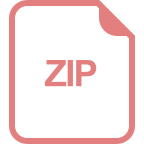















