php 读取word文档中的图片文字和数学公式,并存入数据库
时间: 2024-02-29 09:52:03 浏览: 18
要读取Word文档中的图片、文字和数学公式,并存入数据库,可以使用PHPWord库和PHPDocx库来实现。以下是一个示例代码:
```php
// 导入PHPWord库
require_once 'PHPWord/vendor/autoload.php';
// 导入PHPDocx库
require_once 'phpdocx/vendor/autoload.php';
// 创建PHPWord对象
$phpWord = \PhpOffice\PhpWord\IOFactory::load('path/to/word/document.docx');
// 读取所有文本、图片和数学公式
$allText = '';
$allImages = [];
$allMathMLs = [];
foreach ($phpWord->getSections() as $section) {
foreach ($section->getElements() as $element) {
if ($element instanceof \PhpOffice\PhpWord\Element\Text) {
$allText .= $element->getText() . "\n";
} elseif ($element instanceof \PhpOffice\PhpWord\Element\Image) {
$imageBlob = $element->getImageBlob();
$imageType = $element->getImageType();
$allImages[] = [$imageBlob, $imageType];
} elseif ($element instanceof \PhpOffice\PhpWord\Element\TextRun) {
$mathML = $element->getMathML();
if ($mathML) {
$allMathMLs[] = $mathML;
}
}
}
}
// 存储所有文本、图片和数学公式到数据库
$db = new PDO('mysql:host=localhost;dbname=mydatabase', 'myusername', 'mypassword');
$stmt = $db->prepare('INSERT INTO mytable (text, image, mathml) VALUES (:text, :image, :mathml)');
$stmt->bindParam(':text', $allText);
foreach ($allImages as $imageData) {
$stmt->bindParam(':image', $imageData[0], PDO::PARAM_LOB);
$stmt->execute();
}
foreach ($allMathMLs as $mathML) {
$stmt->bindParam(':mathml', $mathML);
$stmt->execute();
}
```
上述代码中,`path/to/word/document.docx`需要替换为实际的Word文档路径。代码会将文档中的所有文本、图片和数学公式读取到变量`$allText`、`$allImages`和`$allMathMLs`中,并将它们存储到数据库中。注意,存储图片需要将图片数据绑定到PDOStatement对象中,存储数学公式则可以直接绑定字符串。
相关推荐
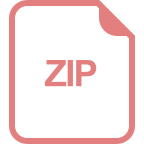














