public int[] twoSum(int[] nums, int target) { Map<Integer, Integer> map = new HashMap<>(); for (int i = 0; i < nums.length; i++) { map.put(nums[i], i); } for (int i = 0; i < nums.length; i++) { int complement = target - nums[i]; if (map.containsKey(complement) && map.get(complement) != i) { return new int[] { i, map.get(complement) }; } } throw new IllegalArgumentException("No two sum solution");
时间: 2024-01-27 22:03:12 浏览: 32
这也是一段 Java 代码,实现了在给定整数数组 nums 中找出两个数,使它们的和为目标数 target,并返回这两个数的下标。
具体实现思路是:先使用哈希表将数组中的元素及其下标存储起来,然后再遍历数组,对于每个元素,计算出它与目标数 target 的差值 complement,检查哈希表中是否存在 complement,如果存在且不是当前元素,则返回当前元素和 complement 的下标。如果遍历完整个数组都没有找到符合条件的两个数,则抛出 IllegalArgumentException 异常。
该算法的时间复杂度为 O(n),空间复杂度为 O(n)。它与第一段代码的区别在于,第一段代码在遍历数组时就已经开始寻找符合条件的两个数,而这段代码先将数组中的元素及其下标存储起来,再遍历数组时再进行查找,会多出一次循环,但是代码更加简洁易懂。
相关问题
public int[] twoSum(int[] nums, int target) { Map<Integer, Integer> map = new HashMap<Integer, Integer>(); for (int i = 0; i < nums.length; i++) { int complement = target - nums[i]; if (map.containsKey(complement)) { return new int[]{map.get(complement), i}; } map.put(nums[i], i); } return new int[] {}; }使用该代码优化完成
这个代码已经很优化了,不过我们可以稍微做一些改进。以下是优化后的代码:
```java
public static int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[]{map.get(complement), i};
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
```
在这个代码中,我们将返回一个抛出异常的语句替换了原来的返回空数组的语句。这样做的好处是,如果没有找到符合条件的结果,程序会立即停止并抛出异常,避免了后续程序可能会出现的空指针异常等问题。
希望这个优化后的代码可以更好地帮助您解决问题!
测试这段代码public int[] twoSum(int[] nums, int target) { Map<Integer, Integer> hashtable = new HashMap<Integer, Integer>(); for (int i = 0; i < nums.length; ++i) { if (hashtable.containsKey(target - nums[i])) { return new int[]{hashtable.get(target - nums[i]), i}; } hashtable.put(nums[i], i); } return new int[0]; }
这段代码是一个方法,需要在一个 Java 类中进行调用测试。您可以将这段代码粘贴到一个 Java 类的方法中,然后在该类的 main 方法中调用它进行测试。以下是一个示例代码:
```java
import java.util.Arrays;
import java.util.Map;
import java.util.HashMap;
public class TwoSumTest {
public static void main(String[] args) {
int[] nums = {2, 7, 11, 15};
int target = 9;
int[] result = twoSum(nums, target);
System.out.println(Arrays.toString(result)); // [0, 1]
}
public static int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> hashtable = new HashMap<Integer, Integer>();
for (int i = 0; i < nums.length; ++i) {
if (hashtable.containsKey(target - nums[i])) {
return new int[]{hashtable.get(target - nums[i]), i};
}
hashtable.put(nums[i], i);
}
return new int[0];
}
}
```
在上面示例代码中,我们将两个测试用例写在了 main 方法中,分别使用了不同的数组和目标值。您可以在您的 Java 开发环境中运行这段代码进行测试,看看结果是否符合预期。
相关推荐
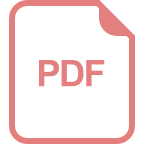
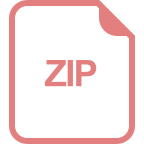
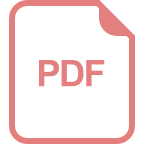













